Swift – Check if Arrays are Equal
Two arrays are said to be equal if they contain the same elements in the same order.
To check if two arrays are equal in Swift, use Equal To ==
operator. Equal To operator returns a Boolean value indicating whether two arrays contain the same elements in the same order. If two arrays are equal, then the operator returns true
, or else it returns false
.
The syntax to use Equal to operator to check if arrays arr1
and arr2
are equal is
arr1 == arr2
Example
In the following program, we take two arrays in arr1
and arr2
. We shall check if these two arrays are equal using equal to operator.
main.swift
var arr1 = ["apple", "mango", "cherry"]
var arr2 = ["apple", "mango", "cherry"]
if (arr1 == arr2) {
print("Two arrays are equal.")
} else {
print("Two arrays are not equal.")
}
Output
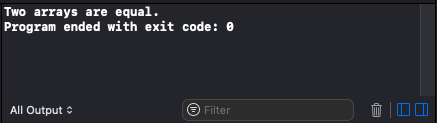
Now let us take elements in the arrays arr1
and arr2
such that the respective elements are not equal.
main.swift
var arr1 = ["apple", "mango", "cherry"]
var arr2 = ["apple", "avacado", "cherry"]
if (arr1 == arr2) {
print("Two arrays are equal.")
} else {
print("Two arrays are not equal.")
}
Output
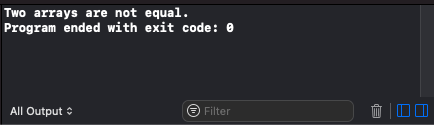
Conclusion
In this Swift Tutorial, we learned how to check if two arrays are equal using Equal to operator.