Swift Function
Swift Function is a logical piece of code which represents a behaviour or functionality. A function can take arguments and can return a value.
Define a Function
To define a function in Swift, use func keyword; followed by function name; followed by the set of parameters that this function can accept, in parenthesis; followed by return type of the function, if any; and then the body of the function enclosed in flower braces.
The syntax of a function in Swift is
func functionName(parameterName1: Type, parameterName2: Type) -> ReturnType {
//function body
}
where parameters and return type are optional.
Examples
In the following example, we will define a function greet
, with no parameters and no return type. This function does print a message. After defining the function, we call it using the function name greet
.
main.swift
func greet() {
print("Hello World")
}
greet()
Output
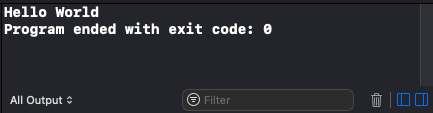
Now, let us modify greet()
, such that is accepts a String for its parameter.
main.swift
func greet(name: String) {
print("Hello \(name)")
}
greet(name: "Abc")
Output
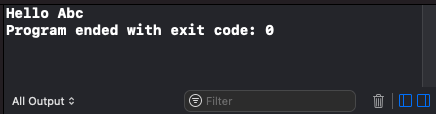
Let us return the message from the function greet()
and then print the returned String value.
main.swift
func greet(name: String) -> String {
return "Hello \(name)"
}
let value = greet(name: "Abc")
print(value)
Output
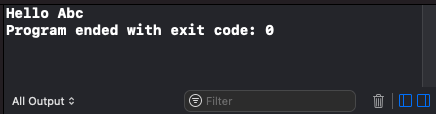
Swift Functions Tutorials
The following tutorials cover some of the concepts or examples related to Swift Functions.
- Swift – Define a Function with Parameters
- Swift – Function that Accepts One or More Values for a Parameter
- Swift – Call Function with no Parameter Labels
- Swift – Custom Label for Function Parameter(s)
- Swift – Assign Default Value for Parameter(s) in Function
- Swift – Pass Parameter by Reference to Function
- Swift – Assign Function to a Variable
- Swift – Pass Function as Parameter
- Swift – Return a Function from another Function
Programs
Conclusion
In this Swift Tutorial, we learned about Functions in Swift programming.