Swift Function – Accept One or More Values for a Parameter
To define a function that can accept one or more values for a parameter, also called a variadic parameter, declare the parameter followed by three period characters ...
after the type.
The syntax to define a function with parameter that can accept one or more values is
func functionName(_ variableName: <T>...) {
//body
}
Please observe the underscore _
followed by a space before parameter declaration, and the three dots ...
after the type in parameter declaration.
Example
In the following example, we will define a function that can accept one or more int values via numbers
parameter and find the sum of these values.
main.swift
func sum(_ numbers: Int...) {
var result = 0
for n in numbers {
result += n
}
print("The sum of numbers is \(result)")
}
sum(2, 3)
sum(1, 2, 8, 4, 6)
Output
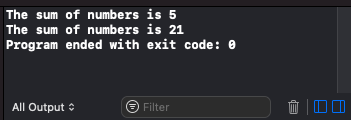
In the first function call, sum(2, 3)
, we passed only two values for the parameter numbers
.
In the second function call, sum(1, 2, 8, 4, 6)
, we passed five values for the parameter numbers
.
We can also not pass any value for the variadic parameter.
main.swift
func sum(_ numbers: Int...) {
var result = 0
for n in numbers {
result += n
}
print("The sum of numbers is \(result)")
}
sum()
Output
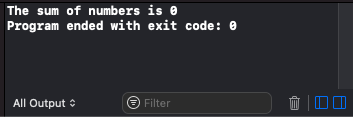
Conclusion
In this Swift Tutorial, we learned how to define a function in Swift with with a variadic parameter that can accept one or more values.