Swift – Pass Function as Parameter
To pass function as parameter to another function in Swift, declare the parameter to receive a function with specific parameters and return type.
The syntax to declare the parameter that can accept a function is same as that of declaring a variable to store a function.
A basic pseudo code to pass function as parameter would be
func functionName(parameterName: (Type, Type) -> ReturnType) -> ReturnType { //body }
Please note that we have specified parameters and return type for the parameter that can accept a function.
Example
In the following program, we will define a calculate()
function that can accept two numbers for parameters a
and b
, and accept a function for the parameter operation
.
main.swift
func addition(a: Int, b: Int) -> Int { return a + b } func subtraction(a: Int, b: Int) -> Int { return a - b } func multiply(a: Int, b: Int) -> Int { return a * b } func division(a: Int, b: Int) -> Int { return a / b } func calculate(a: Int, b: Int, operation: (Int, Int) -> Int) { let result = operation(a, b) print(result) } calculate(a: 7, b: 2, operation: addition) calculate(a: 7, b: 2, operation: subtraction) calculate(a: 7, b: 2, operation: multiply) calculate(a: 7, b: 2, operation: division)
Output
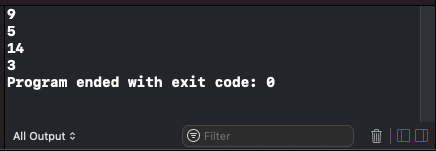
ADVERTISEMENT
Conclusion
In this Swift Tutorial, we learned how to pass a function as parameter to another function in Swift.