Swift – Check if Variable/Object is String
To check if a variable or object is a String, use is
operator as shown in the following expression.
x is String
where x
is a variable/object.
The above expression returns a boolean value: true
if the variable is a String, or false
if not a String.
Example
In the following program we will initialise a String variable, and programmatically check if that variable is a String or not using is
Operator.
main.swift
var x = "Hello World" let result = x is String print("Is the variable a String? \(result)")
Output
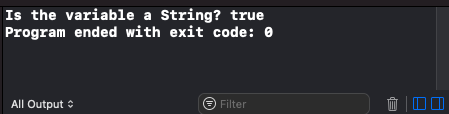
Now, let us take a variable x
of type Int, and check if x
is String or not using is
Operator.
main.swift
var x = 25 let result = x is String print("Is the variable a String? \(result)")
Output
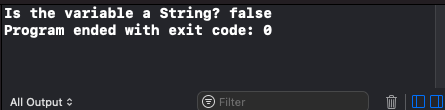
We may use this expression as a condition in if statement.
main.swift
var x = 25 if x is String { print("The variable is a String.") } else { print("The variable is not a String.") }
Output
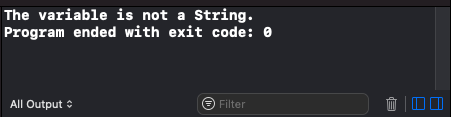
ADVERTISEMENT
Conclusion
In this Swift Tutorial, we learned how to check if a variable or object is of type String or not using is
Operator.