Swift Function – Find Sum of One or More Ints
To write a function that finds the sum of one or more integer values, we can declare a variadic parameter as shown below.
</>
Copy
func sum(_ numbers: Int...) {
//body
}
Please observe the underscore _
followed by a space before parameter declaration, and the three dots ...
after the type in parameter declaration.
Example
In the following example, we will define a function that can accept one or more int values via numbers
parameter and find the sum of these values.
main.swift
</>
Copy
func sum(_ numbers: Int...) {
var result = 0
for n in numbers {
result += n
}
print("The sum of numbers is \(result)")
}
sum(2, 3)
sum(1, 2, 8, 4, 6)
Output
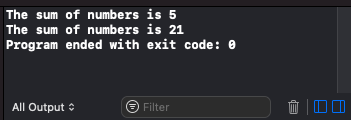
Conclusion
In this Swift Tutorial, we learned how to define a function in Swift to accept one or more int values and find their sum.