Swift Array – While Loop
To iterate over each element of a Swift Array, we may use while loop, increment index from 0 until array length, and access each element during respective iteration.
The following code snippet demonstrates how to use while loop with array, and iterate over each element of the array.
var i = 0 while i < array.count { var element = array[i] //code }
during each iteration, we have access to ith element, where i
increments from 0 until the array length.
Examples
In the following program, we will take a string array, fruits
, and iterate over each of the element using while loop.
main.swift
var fruits:[String] = ["Apple", "Banana", "Mango"] var i = 0 while i < fruits.count { let fruit = fruits[i] //access ith element print(fruit) i += 1 }
Output
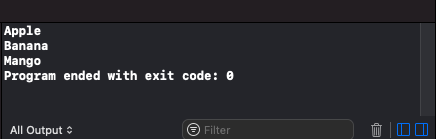
Now, let us take an array of integers, and iterate over this array using while loop.
main.swift
var primes:[Int] = [2, 3, 5, 7, 11] var i = 0 while i < primes.count { let prime = primes[i] print(prime) i += 1 }
Output
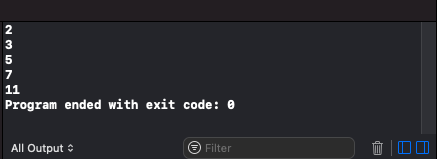
ADVERTISEMENT
Conclusion
Concluding this Swift Tutorial, we learned how to iterate over array elements using while loop in Swift.