Swift Array – With Elements of Different Types
In Swift, we can define an array that can store elements of any type. These are also called Heterogenous Collections.
To define an array that can store elements of any type, specify the type of array variable as [Any].
</>
Copy
var arr: [Any]
We can also infer the array variable/literal to [Any] as shown in the following example.
</>
Copy
var arr = [element_1, element_2,..] as [Any]
Now, let us go through some examples, where we define and initilize an Array with elements of different types.
Examples
In the following example, we will define an array arr
with elements of type Integer, String and Float.
main.swift
</>
Copy
var arr = [2, "ac", "abc", 5, 3.6] as [Any]
print("Array : \(arr)")
Output
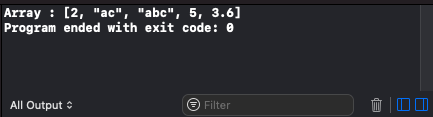
Now, let us print the type of this array variable.
main.swift
</>
Copy
var arr: [Any] = [2, "ac", "abc", 5, 3.6]
print(type(of: arr))
Output
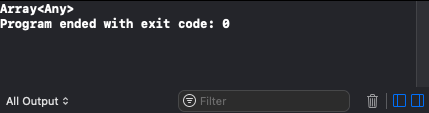
The array is of type Array<Any>.
Conclusion
In this Swift Tutorial, we learned how to define an initialize an Array with elements belonging to different types.