Swift Addition Operator
In Swift, Arithmetic Addition Operator is used to find the sum of given two numbers.
In this tutorial, we will learn about Addition Operator in Swift language, its syntax, and how to use this Addition Operator in programs, with examples.
Syntax
The syntax of Addition Operator is
x + y
where
Operand / Symbol | Description |
---|---|
x | Left operand. A numeric value. |
+ | Symbol for Addition Operator. |
y | Right operand. A numeric value. |
The expression x + y
returns a numeric value representing the sum of x and y.
Examples
1. Addition of integers
In the following program, we take two integer values in variables: x and y, and find their sum using Addition Operator.
main.swift
var x = 5
var y = 2
var result = x + y
print("\(x) + \(y) is \(result)")
Output
5 + 2 is 7
2. Addition of float values
In the following program, we take two floating point values in variables: x and y, and find their sum using Addition Operator.
main.swift
var x: Float = 5.0
var y: Float = 2.3
var result = x + y
print("\(x) + \(y) is \(result)")
Output
5.0 + 2.3 is 7.3
3. Add values of different datatypes
If we try to add two numbers of different types, we would get Swift Compiler Error as shown below.
main.swift
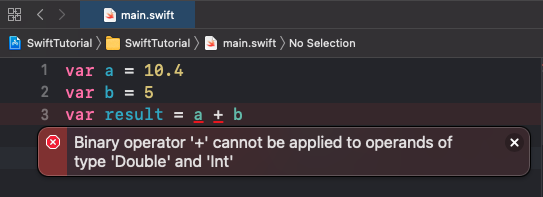
Summary
In this Swift Operators tutorial, we learned about Arithmetic Addition Operator, its syntax, and usage, with examples.