Swift – Convert String to Int
To convert a String value to Integer value in Swift, use Int() and Optional Value unwrapping.
The syntax to convert a String x
to Integer is
</>
Copy
Int(x)
Int()
returns an Optional Integer value, and we can convert this to an Integer value using Optional Value Unwrapping !
, as shown in the following.
</>
Copy
var result = Int(str)
if result != nil {
let x = result! //unwrap to Int
//code
}
Examples
In this example, we take a String value in str
and convert this value to Integer.
main.swift
</>
Copy
var str = "256"
var result = Int(str)
if result != nil {
let x = result! //unwrap to Int
print(x)
}
Output
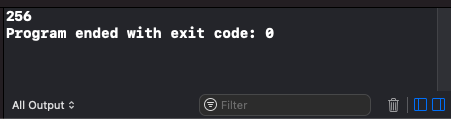
If the value in String cannot be converted to an Integer, Int()
returns nil
value. In that case, check if the value returned by Int()
is not nil
. Only if not nil
, proceed with unwrapping the returned optional value.
main.swift
</>
Copy
var str = "256 abc"
var result = Int(str)
if result != nil {
let x = result! //unwrap to Int
print(x)
} else {
print("Result of Int() is nil.")
}
Output
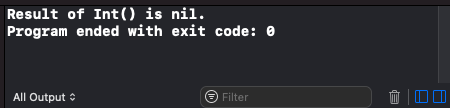
Conclusion
In this Swift Tutorial, we have learned how to convert a String to Integer in Swift programming.