Swift – Insert Another Array in Array at Specific Index
To insert another Array in Swift Array at specific index, call insert(contentsOf:at:)
method. Pass the other array as value for contentsOf
and the index as as value for at
.
insert(contentsOf:at:)
method inserts the content of other array at the specified index.
The syntax to insert contents of another array to this array at specific index is
arrayName.insert(contentsOf: anotherArray, at:index)
We can only insert a contents of array whose type is same as that of the elements in this array. Also, the index has to be within the index limits of the array.
Examples
In the following program, we will take an array fruits
, and insert contents of another array moreFruits
in this fruits
array at index 2
.
main.swift
var fruits = ["apple", "banana", "cherry"] var moreFruits = ["mango", "guava"] var index = 2 fruits.insert(contentsOf:moreFruits, at:index) print(fruits)
Output
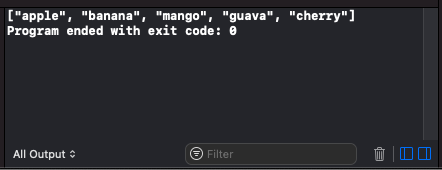
The contents of of moreFruits
has been inserted in fruits
array at specified index 2
.
The index at which we would like insert should be within the index range of the array. Otherwise, we would get runtime error.
Let us try to insert an array at index 8
, when the array length is only 3
. We get runtime error as shown in the following example.
main.swift
var fruits = ["apple", "banana", "cherry"] var moreFruits = ["mango", "guava"] var index = 8 fruits.insert(contentsOf:moreFruits, at:index) print(fruits)
Output
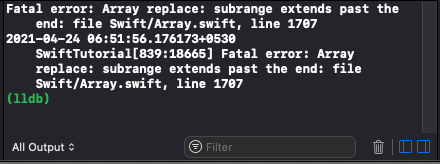
Conclusion
In this Swift Tutorial, we learned how to insert contents of another array in this array, at specified index.