Swift Subtraction Operator
To perform subtraction of two numbers in Swift, we can use Swift Subtraction Arithmetic operator.
Subtraction Operator -
takes two numbers as operands and returns the difference of second operand from the first operand.
Syntax
The syntax of Subtraction Operator is
a - b
where a
and b
are left and right operands respectively.
These operands should be of same type.
For example,
a
andb
can be Int.a
andb
can be Float.a
andb
can be Double.
Examples
1. Subtraction of integer values
In the following program, we will perform subtraction of two integers.
main.swift
var a = 10
var b = 4
var result = a - b
print("The result is \(result)")
Output
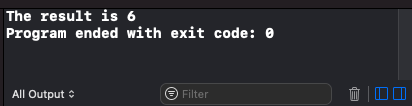
2. Subtraction of Double values
Now, let us find the subtraction of two Double values.
main.swift
var a = 10.2
var b = 4.1
var result = a - b
print("The result is \(result)")
Output
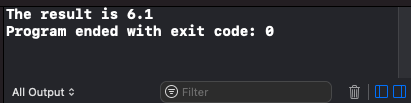
2. Subtraction with values of different datatypes
If we try to subtract numbers of different types, we would get Swift Compiler Error as shown below.
main.swift
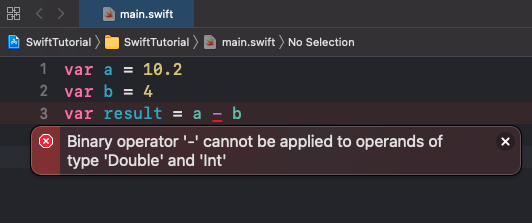
Since a
is Double and b
is Int, Subtraction -
operator raises Compiler Error.
Conclusion
In this Swift Tutorial, we learned how to perform subtraction in Swift programming using Subtraction operator. We have seen the syntax and usage of Subtraction operator, with examples.