Swift – Insert an Element in Array at Specific Index
To insert an element in Swift Array at specific index, call insert(_:at:)
method. Pass the element as first argument and the index as value for at
parameter.
insert(_:at:)
method inserts the given element (first argument) at specified index.
The syntax of insert(_:at:)
method that inserts an element to the array at the index is
arrayName.insert(element, at:index)
We can only insert an element whose type is same as that of the elements in the array. Also, the index has to be within the index limits of the array.
Examples
In the following program, we will take an array fruits
, and insert an element "guava"
to this fruits
array at index 2
.
main.swift
var fruits = ["apple", "banana", "cherry", "mango"]
var anotherFruit = "guava"
var index = 2
fruits.insert(anotherFruit, at:index)
print(fruits)
Output
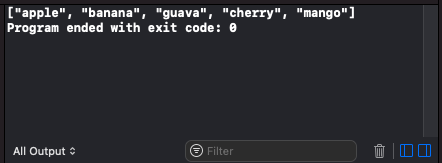
The element anotherFruit
is inserted in fruits
array at specified index 2
.
The index that we would like insert the element should be within the index range of the array. Otherwise, we would get runtime error.
Let us try to insert an element at index 8
, when the array length is only 4
.
main.swift
var fruits = ["apple", "banana", "cherry", "mango"]
var anotherFruit = "guava"
var index = 8
fruits.insert(anotherFruit, at:index)
print(fruits)
Output
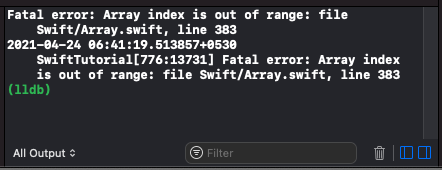
Conclusion
In this Swift Tutorial, we learned how to insert an element in array, at specified index.