Swift – Check if Set is Empty
To check if a Set is empty in Swift, we can check the Set’s isEmpty
property or count
property.
Set.isEmpty
property returns a boolean value of true
if the Set is empty, or false
if the Set is not empty.
Set.count
property returns zero if the Set is empty, or a value greater than zero if the Set is not empty.
In this tutorial, we will go through examples covering these two ways.
Examples
In the following program, we will take an empty Set and check programmatically if the Set is empty or not, using isEmpty property of this Set.
main.swift
var x = Set<Int>()
if x.isEmpty {
print("Set is empty.")
} else {
print("Set is not empty.")
}
Output
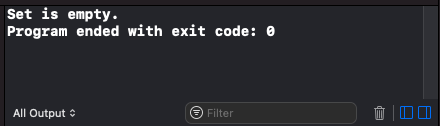
Now, let us use count property and check if Set is empty or not.
main.swift
var x = Set<Int>()
if x.count == 0 {
print("Set is empty.")
} else {
print("Set is not empty.")
}
Output
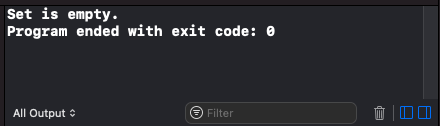
Let us take a Set with some values and check if it is empty.
main.swift
var x: Set = [1, 4, 9]
if x.isEmpty {
print("Set is empty.")
} else {
print("Set is not empty.")
}
Output
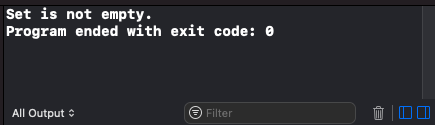
Conclusion
In this Swift Tutorial, we have learned how to check if a Set is empty or not.