Swift – Filter String Array based on String Length
To filter strings in a Swift String Array based on length, call filter()
method on this String Array, and pass the condition prepared with the string length as argument to the filter()
method.
filter() method returns an array with only those elements that satisfy the given predicate/condition.
Example
In the following program, we will take an array of Strings, and filter those elements whose string length is greater than 5
.
main.swift
var arr = ["a", "bbbbbb", "ccc", "dd", "eeeeee"]
let result = arr.filter { $0.count > 5 }
print("Original Array : \(arr)")
print("Filtered Array : \(result)")
Output
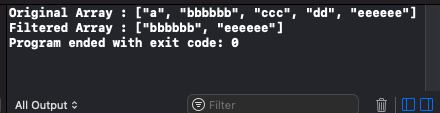
Only those strings whose length is greater than five, made it to the resulting array.
Let us now filter those strings whose length is less than 5
.
main.swift
var arr = ["a", "bbbbbb", "ccc", "dd", "eeeeee"]
let result = arr.filter { $0.count > 5 }
print("Original Array : \(arr)")
print("Filtered Array : \(result)")
Output
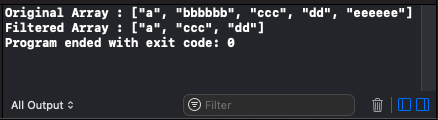
Only those strings whose length is less than five, made it to the resulting array.
Conclusion
Concluding this Swift Tutorial, we learned how to filter those strings whose length satisfies the given condition.