Swift Multiplication Operator
To perform multiplication of two numbers in Swift, we can use Swift Multiplication Arithmetic operator.
Multiplication Operator *
takes two numbers as operands and returns the product of the two operands.
Syntax
The syntax of Multiplication Operator is
a * b
where a
and b
are left and right operands respectively.
These operands should be of same type. For example,
a
andb
can be Int.a
andb
can be Float.a
andb
can be Double, etc.
Examples
1. Multiplication of integer values
In the following program, we will perform multiplication of two integers.
main.swift
var a = 3
var b = 4
var result = a * b
print("The result is \(result)")
Output
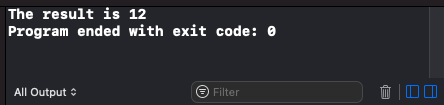
2. Multiplication of Double values
Now, let us find the multiplication of two Double values.
main.swift
var a = 3.2
var b = 4.1
var result = a * b
print("The result is \(result)")
Output
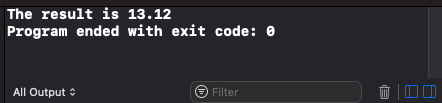
3. Multiplication of values that belong to different datatypes
If we try to multiply numbers of different types, we would get Swift Compiler Error as shown below.
main.swift
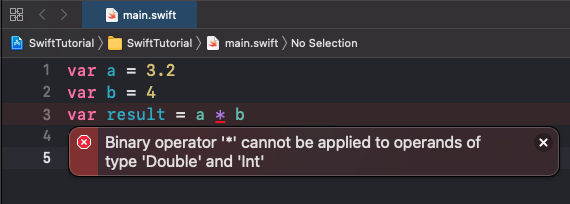
Since a
is Double and b
is Int, Multiplication -
operator raises Compiler Error.
Conclusion
In this Swift Tutorial, we learned how to perform Multiplication in Swift programming using Multiplication Operator. We have seen the syntax and usage of Multiplication Operator with examples.