Swift – Return a Function from another Function
To return a Function b
from another Function a
in Swift, specify the complete parameters and return type of function b
as return type of the function a
.
The return type of function a
is formed from the parameters and return type of function b
.
The syntax to return a function b
from function a
is
</>
Copy
func a(parameters) -> (Function_b_Parameters_Type) -> Function_b_ReturnType {
func b (someParameters) -> ReturnType {
//body
}
}
Examples
In the following program, we will return addition() function from calculate() function.
main.swift
</>
Copy
func calculate() -> (Int, Int) -> Int {
func addition(a: Int, b: Int) -> Int {
return a + b
}
return addition
}
//variable to store function
var myFunc: (Int, Int) -> Int
myFunc = calculate()
print("Addition")
print(myFunc(2, 5))
In the following program, we will return any of the function addition()
, subtraction()
, multiplication()
or division()
, from function calculate()
based on parameter passed to calculate()
function.
main.swift
</>
Copy
func calculate(operation: String) -> (Int, Int) -> Int {
func addition(a: Int, b: Int) -> Int {
return a + b
}
func subtraction(a: Int, b: Int) -> Int {
return a - b
}
func multiply(a: Int, b: Int) -> Int {
return a * b
}
func division(a: Int, b: Int) -> Int {
return a / b
}
switch operation {
case "+":
return addition
case "-":
return subtraction
case "*":
return multiply
case "/":
return division
default:
return addition
}
}
//variable to store function
var myFunc: (Int, Int) -> Int
myFunc = calculate(operation: "+")
print("Addition")
print(myFunc(2, 5))
myFunc = calculate(operation: "-")
print("Subtraction")
print(myFunc(2, 5))
myFunc = calculate(operation: "*")
print("Multiplition")
print(myFunc(2, 5))
myFunc = calculate(operation: "/")
print("Division")
print(myFunc(2, 5))
Output
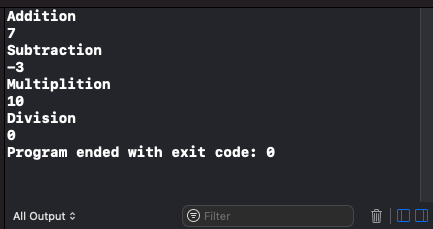
Conclusion
In this Swift Tutorial, we learned how to return a function from another function in Swift.