Swift Array – For Loop
To iterate over each element of a Swift Array, we can use for loop, and access each element during respective iteration.
The following code snippet demonstrates how to use for loop with array, and iterate over each element of the array.
for element in array { //code }
where array
is the array of elements, and element
contains that element of the array during that iteration.
Examples
In the following program, we will take a string array, fruits
, and iterate over each of the element using for loop.
main.swift
var fruits:[String] = ["Apple", "Banana", "Mango"] for fruit in fruits { print(fruit) }
Output
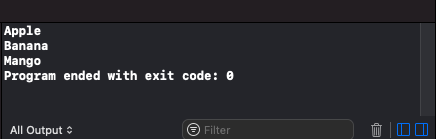
Now, let us take an array of integers, and iterate over this array using for loop.
main.swift
var primes:[Int] = [2, 3, 5, 7, 11] for prime in primes { print(prime) }
Output
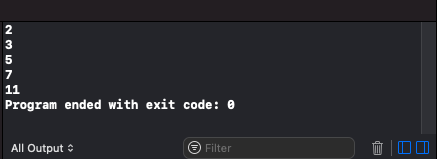
ADVERTISEMENT
Conclusion
Concluding this Swift Tutorial, we learned how to iterate over array elements using for loop in Swift.