Swift – Access Elements of Array using Index
To access elements of a Swift Array using Index, use the index as subscript on the array variable.
The syntax to access element of an array at specific index is
var element = arrayName[index]
where
arrayName
is the array from which we would like to access the element, andindex
is the integer which represents the position of element in the array.
The index starts at 0
, and increments in steps of 1
until the length of the array. Which means the first element of a nonempty array is always at index zero
A negative index or an index equal to or greater than array length triggers a runtime error.
Examples
In the following program, we will create an array with file elements and get the elements at index 1 and 3 respectively.
main.swift
var fruits: [String] = ["apple", "banana", "cherry", "mango", "guava"] //element at index = 1 let element_1 = fruits[1] print("Element at index=1 is \(element_1)") //element at index = 3 let element_3 = fruits[3] print("Element at index=3 is \(element_3)")
Output
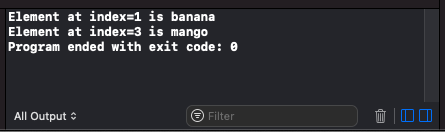
Negative Index
If we try to access an array with negative number as index, we get runtime error, as shown in the following program.
main.swift
var fruits: [String] = ["apple", "banana", "cherry", "mango", "guava"] //element at index = -2 let element_1 = fruits[-2] print("Element at index=-2 is \(element_1)")
Output
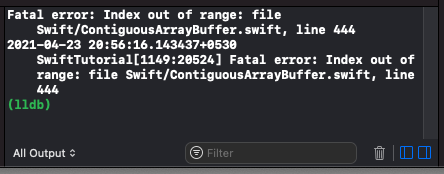
Index >= Array Length
If we try to access an array with an index greater than the array length, we get runtime error, as shown in the following program.
main.swift
var fruits: [String] = ["apple", "banana", "cherry", "mango", "guava"] //element at index = 8 let element_1 = fruits[8] print("Element at index=8 is \(element_1)")
Output
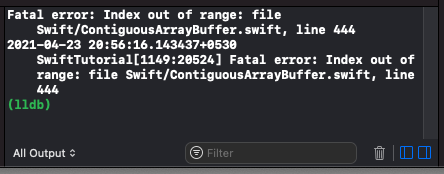
Conclusion
In this Swift Tutorial, we learned how to access elements of an array using index.