Swift Subtraction Assignment Operator
In Swift, Subtraction Assignment Operator is used to compute the difference of given value in right operand from the value in left operand, and assign the result back to the variable given as left operand.
In this tutorial, we will learn about Subtraction Assignment Operator in Swift language, its syntax, and how to use this Assignment Operator in programs, with examples.
Syntax
The syntax of Subtraction Assignment Operator is
x -= value
where
Operand / Symbol | Description |
---|---|
x | Left operand. A variable. |
-= | Symbol for Subtraction Assignment Operator. |
value | Right operand. A value, an expression that evaluates to a value, a variable that holds a value, or a function call that returns a value. |
The expression x -= value
computes x – value and assigns this computed value to variable x.
Therefore the expression x -= value
is same as x = x - value
.
Examples
1. Subtraction Assignment of integer value to x
In the following program, we shall Subtract assign an integer value of 25 to variable x using Subtraction Assignment Operator.
main.swift
var x = 70 x -= 25 print("x is \(x)")
Output
x is 45
Explanation
x -= 25 x = x - 25 = 70 - 25 = 45
2. Subtraction Assignment of an expression to x
In the following program, we shall subtract and assign the value of an expression to variable x using Subtraction Assignment Operator.
main.swift
var x = 40 var y = 5 x -= (2 + y) * 4 print("x is \(x)")
Output
x is 12
Explanation
x -= (2 + y) * 4 x = x - (2 + y) * 4 = 40 - (2 + 5) * 4 = 40 - 28 = 12
3. Subtract Assignment with strings
We cannot use Subtraction assignment operator with string values.
main.swift
var x = "Hello " x -= "Ram" print("x is \"\(x)\"")
Output
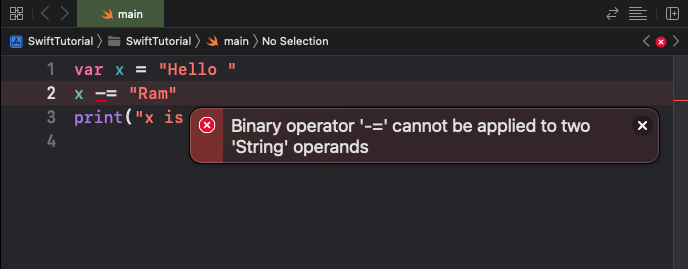
Summary
In this Swift Operators tutorial, we learned about Subtraction Assignment Operator, its syntax, and usage, with examples.