Swift – Find Largest Number in Array
To find the largest number in an array in Swift, call max() method. max() method returns the maximum of the elements present in the array.
The syntax to call max() method on the array is
</>
Copy
var largest = arr.max()
max() method returns object of type Optional<T>. We may type cast this value to required datatype.
Example
In the following program, we will initialize an array with numbers, and find the largest number of this array using max() method.
main.swift
</>
Copy
var arr = [1, 99, 6, 74, 5]
var largest = arr.max()
print("Largest number in this array is \(largest)")
Output
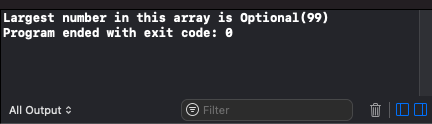
Now, we can unwrap the value in largest using let
.
main.swift
</>
Copy
var arr = [1, 99, 6, 74, 5]
let largest = arr.max()
switch largest {
case .some(let unwrapped):
print("Largest number in this array is: \(unwrapped)")
case .none:
print("nil")
}
Output
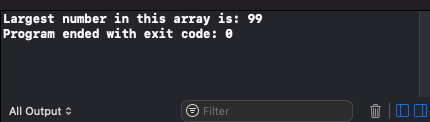
Conclusion
In this Swift Tutorial, we learned how to find the largest number in a given numeric array using Array.max() method.