In this tutorial, you will learn how to check if a file exists at given file path in Swift language using fileExists() method.
Check if a File Exists in Swift
To check if a file exists by a given path in Swift, follow these steps.
- Given a path to the file.
- Get the default shared File manager using FileManager.defaut.
- Call fileExists() method on the file manager object, and pass the file path as argument.
- If a file exists at given the given path, then fileExists() method returns a boolean value of true.
- Or if a file does not exist at given the given path, then fileExists() method returns a boolean value of false.
- You can use a Swift if-else statement with the value returned by fileExists() method as condition.
Examples
1. Check if file at given path exists [Positive Scenario]
In this example, we take a file path "/Users/tutorialkart/data.txt"
, and check if a file exists by the given path, using fileExists() method.

We have taken the file path such that the file exists. Therefore, the fileExists() method should return true, and the if-block should execute.
main.swift
import Foundation let path = "/Users/tutorialkart/data.txt" let fileManager = FileManager.default if fileManager.fileExists(atPath: path) { print("FILE EXISTS.") } else { print("FILE DOES NOT EXIST.") }
Output
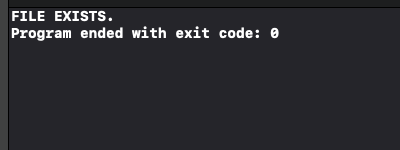
2. Check if file at given path exists [Negative Scenario]
In this example, we take a file path "/Users/tutorialkart/dummy.txt"
, and check if a file exists by the given path, using fileExists() method.
We have take the file path such that the file does not exist. Therefore, the fileExists() method should return false, and the else-block should execute.
main.swift
import Foundation let path = "/Users/tutorialkart/dummy.txt" let fileManager = FileManager.default if fileManager.fileExists(atPath: path) { print("FILE EXISTS.") } else { print("FILE DOES NOT EXIST.") }
Output
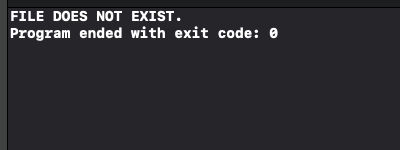
Conclusion
In this Swift Tutorial, we have seen how to check if a file exists at given path, using fileExists() method, with examples.