In this tutorial, you will learn how to check if a directory exists in Swift language using fileExists() method.
Check if given Path is a Directory in Swift
To check if given directory exists or not, using Swift, we have to check if given path exists, and if it exists, check if it is a directory at given path.
Follow these steps.
- Given a path.
- Get the default shared File manager using FileManager.defaut.
- Take a boolean variable of type ObjCBool, and initialise it to false, say isDir.
- Call fileExists() method of the file manager, and pass the path and boolean variable isDir as arguments.
- If the given path is a directory, then the given boolean value isDir is set to true. And the fileExists() returns true if the directory exists. We combine these two conditions using Swift AND logical operator, and use it as a condition in Swift if-else statement.
The syntax to call fileExists() method to check if given path exists, and check if the path is a directory, in total to check if directory exists is
fileExists(atPath: path, isDirectory: &isDir) && isDir.boolValue
Examples
1. Check if directory exists at given path
In this example, we take a path "/Users/tutorialkart/"
, and check if this directory exists or not.
main.swift
import Foundation
let path = "/Users/tutorialkart/"
let fileManager = FileManager.default
var isDir: ObjCBool = false
if fileManager.fileExists(atPath: path, isDirectory: &isDir) && isDir.boolValue {
print("Given DIRECTORY exists.")
} else {
print("Given DIRECTORY does not exist.")
}
Output
We have taken the path such that the path is a directory and the directory exists. Therefore, we get the following result.
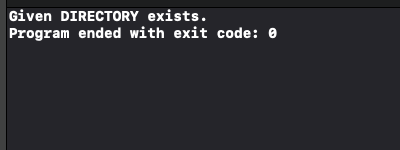
2. Directory does not exist at given path
In this example, we take a path "/Users/tutorialkart/dummy/"
, and check if a directory exists by this given path.
main.swift
import Foundation
let path = "/Users/tutorialkart/dummy/"
let fileManager = FileManager.default
var isDir: ObjCBool = false
if fileManager.fileExists(atPath: path, isDirectory: &isDir) && isDir.boolValue {
print("Given DIRECTORY exists.")
} else {
print("Given DIRECTORY does not exist.")
}
Output
The given path does not exist and therefore fileExists() returns False. Else-block is executed.
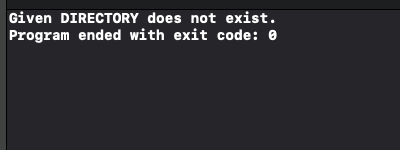
Conclusion
In this Swift Tutorial, we have seen how to check if a directory exists or not, using fileExists() method, with examples.