Swift – Check if Variable/Object is Int
To check if a variable or object is an Int, use is
operator as shown in the following expression.
x is Int
where x
is a variable/object.
The above expression returns a boolean value: true
if the variable is an Int, or false
if not an Int.
Example
In the following program we will initialise an Integer variable, and programmatically check if that variable is an Int or not using is
Operator.
main.swift
var x = 41
let result = x is Int
print("Is the variable an Int? \(result)")
Output
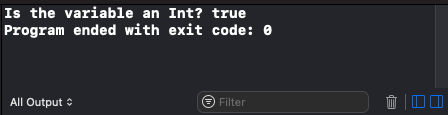
Now, let us take a variable x
of type String, and check if x
is Int or not using is
Operator. Since, x
is not an Int, the expression x is Int
should return false.
main.swift
var x = "Hello World"
let result = x is Int
print("Is the variable an Int? \(result)")
Output
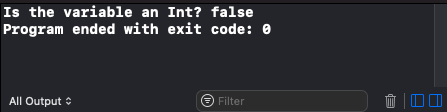
We may use this expression as a condition in if statement.
main.swift
var x = 25
if x is Int {
print("The variable is an Int.")
} else {
print("The variable is not an Int.")
}
Output
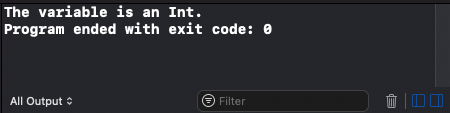
Conclusion
In this Swift Tutorial, we learned how to check if a variable or object is of type Int or not using is
Operator.