Swift defer
Swift defer
keyword is used to execute a block of code at a later point in time, which is just before the end of execution of current scope, where the scope is defer statement’s scope.
The syntax to use defer keyword is
defer {
//code
}
The code inside defer block executes only at the end of the execution of this defer’s scope.
Examples
In the following program, we define defer code in which there is a print statement. The scope of this defer statement is the scope of the program. So, this defer statement will execute at the end of the program.
main.swift
print("Hello World")
defer {
print("Bye!")
}
print("Welcome!")
Output
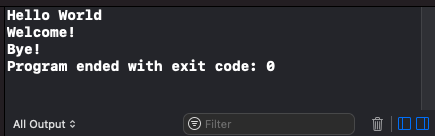
If there are more than one defer blocks in current scope, those defer blocks will execute in order from top to bottom at the end of execution of current scope.
main.swift
print("Hello World")
defer {
print("Bye!")
}
print("Welcome!")
defer {
print("Catch you later!")
}
print("Please, take a seat.")
Output
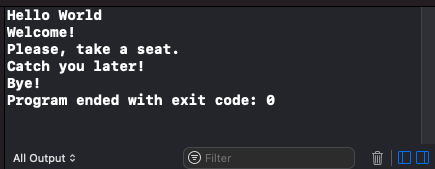
Now, let us write a defer statement inside a function, as shown in the following program. The scope of this defer statement is the function.
main.swift
func greetings() {
print("Hello World")
defer {
print("Bye!")
}
print("Welcome!")
defer {
print("Catch you later!")
}
print("Please, take a seat.")
}
print("Starting program..")
greetings()
print("Ending program..")
Output
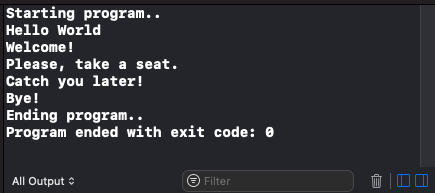
Conclusion
In this Swift Tutorial, we learned how to use defer keyword in Swift, to execute some code at the end of its scope.