Swift guard
Swift guard keyword is used to define a condition, and make sure that the control continues with the execution of the rest of the statements in scope if and only if the condition is true.
If this guard condition is false, then guard-else block is executed in which the control has to be returned from this scope.
The pseudo syntax to use guard statement in Swift is
guard condition else {
//some code
return or throw statement
}
return or throw statement ensures that the control does not fall through, and exits the scope.
Example
In the following program, we will write a function to find the division of two numbers a/b
and guard the condition that b
is not zero.
main.swift
func divide(a: Int, b: Int) {
guard b != 0 else {
print("The denominator cannot be zero.")
return
}
print("\(a) / \(b) = \(a/b)")
}
divide(a: 7, b: 0)
Output
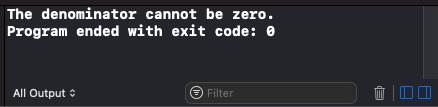
If no return statement is given inside guard else block, Swift causes Compiler Error.
main.swift
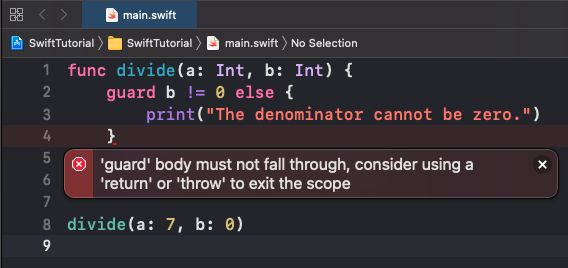
If the guard condition fails, then the statements after guard statements should not execute, and therefore a return statement or throw statement is required to exit the scope.
Conclusion
In this Swift Tutorial, we learned how to use guard statement in Swift with example programs.