In this tutorial, you will learn explore how to write a Swift program to determine whether a given number is a prime number or not.
Swift Program – Prime Number
A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself.
Examples for Prime numbers are 2, 3, 5, 7, 11, 13, 17, 19, 23, etc.
How to check if given number is Prime number
To check if a number is prime, we’ll follow these steps:
- Iterate from 2 to the square root of the number.
- Check if the number is divisible by any of the integers within the iteration range.
- If the number is divisible, it’s not a prime number. Otherwise, it is a prime number.
When we are building the while loop condition, we take advantage of the mathematical fact that if there is no number less than i
that is not a factor then there is no number greater than n/i
that is a factor.
Program
Let’s write a Swift program to determine whether a given number is prime or not. Here’s the program.
main.swift
import Foundation
func isPrime(_ number: Int) -> Bool {
var n = number
if n <= 1 {
return false
}
var i = 2
while i <= n/i {
if n % i == 0 {
return false
}
i += 1
}
return true
}
print("Enter a number : ", terminator: "")
if let number = Int(readLine()!) {
if isPrime(number) {
print("\(number) is a prime number")
} else {
print("\(number) is not a prime number")
}
}
isPrime()
function takes a number and returns a boolean value representing whether the given number is a Prime or not.
Output
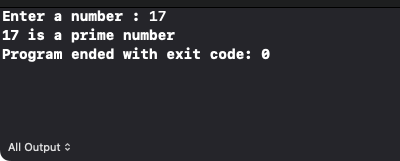
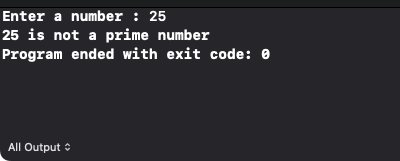
Conclusion
In this Swift Tutorial, we learned how to determine if a given number is a Prime number using Swift. We used While loop to check if there is a factor other than 1 and given number, between 2 and the given number.