In this tutorial, you will learn how to check if a given number is an Armstrong number using Swift programming language.
Swift Program – Armstrong Number
An Armstrong number is a number that is equal to the sum of its own digits raised to the power of the number of digits.
First few Armstrong numbers are: 1, 2, 3, 4, 5, 6, 7, 8, 9, 153, 370, 371, 407, 1634, 8208, 9474, 54748, 92727, 93084, 548834, etc.
How to check if given number is Armstrong number
To check if a number is an Armstrong number, follow these steps:
- Extract the individual digits of the number.
- Calculate the sum of each digit raised to the power of the total number of digits.
- Compare the sum with the original number. If they are equal, then the number is an Armstrong number, otherwise, not an Armstrong number.
Program
Let’s take an example and write a Swift program to check if a given number is an Armstrong number or not. Here’s the program.
main.swift
import Foundation
func isArmstrongNumber(_ number: Int) -> Bool {
var sum = 0
var temp = number
let numberOfDigits = String(number).count
while temp > 0 {
let digit = temp % 10
sum += Int(pow(Double(digit), Double(numberOfDigits)))
temp /= 10
}
return sum == number
}
let numberToCheck = 153
if isArmstrongNumber(numberToCheck) {
print("\(numberToCheck) is an Armstrong number")
} else {
print("\(numberToCheck) is not an Armstrong number")
}
Output
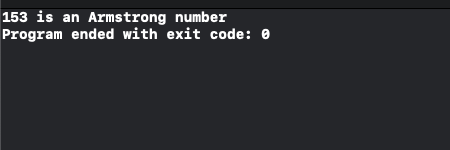
Explanation
Given number = 153
Sum = 13 + 53 + 33
= 1 + 125 + 27
= 153
Given number == Sum
Now, let us take another number, say 812, and check if this number is an Armstrong number.
main.swift
import Foundation
func isArmstrongNumber(_ number: Int) -> Bool {
var sum = 0
var temp = number
let numberOfDigits = String(number).count
while temp > 0 {
let digit = temp % 10
sum += Int(pow(Double(digit), Double(numberOfDigits)))
temp /= 10
}
return sum == number
}
let numberToCheck = 153
if isArmstrongNumber(numberToCheck) {
print("\(numberToCheck) is an Armstrong number")
} else {
print("\(numberToCheck) is not an Armstrong number")
}
Output
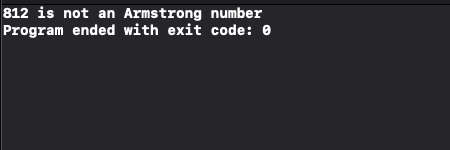
Explanation
Given number = 812
Sum = 83 + 13 + 23
= 512 + 1 + 8
= 521
Given number != Sum
Conclusion
In this Swift Tutorial, we learned how to determine if a given number is an Armstrong number using Swift. We used the concept of extracting individual digits and calculating the sum of digits raised to the power of the total number of digits.