Remove prefix from a string in Swift
To remove a specific prefix from a string in Swift, first check if the string has specified prefix, and if yes, get the count of the characters in the prefix string, and drop that many characters from the original string.
For example, if str is the given string, and we would to remove a specific prefix string prefix, then the syntax would be
var result = str if (str.hasPrefix(prefix)) { result = String(str.dropFirst(prefix.count)) }
The result shall contain the resulting string. If the string str starts with the prefix string, then result is assigned with the string prefix removed. If the string str does not start with the specified prefix string, then the result string shall be same as that of the original string str.
Examples
1. Remove prefix string “hello” from given string
In this example, we will take a string value in str, and remove the prefix string "hello"
if the string starts with the specified prefix string.
main.swift
import Foundation let str = "helloworld" let prefix = "hello" var result = str if (str.hasPrefix(prefix)) { result = String(str.dropFirst(prefix.count)) } print(result)
Output
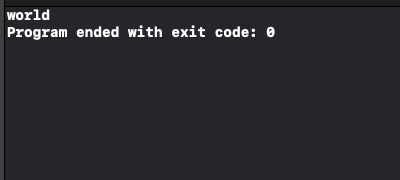
If in case, the string does not start with specified prefix string, then the result string would be same as the original string str.
Conclusion
In this Swift Tutorial, we have seen how to remove a specific prefix string from the given string using dropFirst() method of String class instance.