Repeat a string for N times in Swift
To repeat a given string for specific number of times, say N, in Swift, use the string initialiser with repeating and count parameters.
For example, if str is the given string, and we would to repeat this string for N number of times, then use the following syntax.
</>
Copy
String(repeating: str, count: N)
The above code snippet returns a new string created by repeating the string str for N times.
Examples
1. Repeat string “apple” for 5 times
In this example, we will take a string value in str, say "apple"
, and repeat this string for 5 times.
main.swift
</>
Copy
import Foundation
let str = "apple"
let N = 5
let resultStr = String(repeating: str, count: N)
print(resultStr)
Output
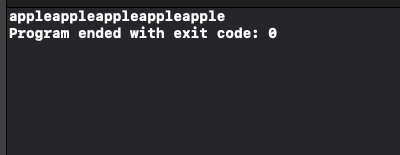
2. Repeat string “h” for 4 times
In this example, we will take a string value in str, say "h"
, and repeat this string for 4 times.
main.swift
</>
Copy
import Foundation
let str = "h"
let N = 4
let resultStr = String(repeating: str, count: N)
print(resultStr)
Output
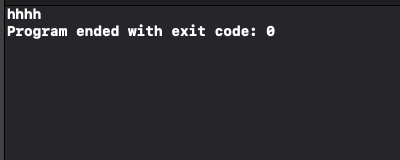
Conclusion
In this Swift Tutorial, we have seen how to repeat a given string for specific number of times using String initializer.