Replace character in string in Swift
To replace all the occurrences of a specific character in a given string with a replacement character in Swift, you can use replacingOccurrences() method of String instance.
Call replacingOccurrences() method on the given string object, and pass the search character for the of parameter, and replacement character for the with parameter.
For example, if you would like to replace the character "x"
with "y"
in a given string str, use the following syntax.
originalString.replacingOccurrences(of: String("x"), with: String("y"))
The above method returns a new string created by replaced character "x"
with character "y"
in the string.
Examples
1. Replace character “o” with “X” in given string
In this example, we take a string value in originalString, and replace the search character "o"
with the replacement character "X"
.
main.swift
import Foundation
var originalString = "Hello, World!"
let searchCharacter: Character = "o"
let replacementCharacter: Character = "X"
let modifiedString = originalString.replacingOccurrences(of: String(searchCharacter), with: String(replacementCharacter))
print("Original String : \(originalString)")
print("Modified String : \(modifiedString)")
Output
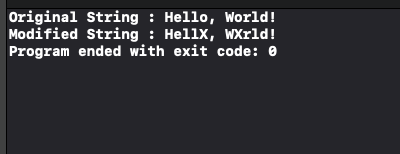
All the occurrences of the search character are replaced with the replacement character in the given string.
Conclusion
In this Swift Tutorial, we have seen how to replace a character in a given string with a replacement character, with examples.