Android Button Text Size
To set Android Button font/text size, we can set android:textSize attribute for Button in layout XML file.
To programmatically set or change Android Button font/text size, we can pass specified size to the method Button.setTextSize(specific_size).
In this tutorial, we will learn how to change the font size of text in Android Button, with the help of example application.
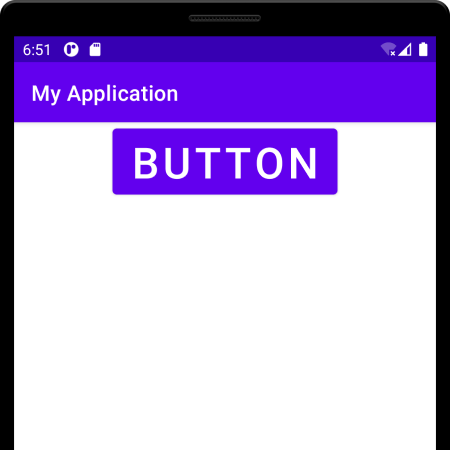
Set Android Button Text Size in Layout File
In this example project, we will change the Button text size in layout file using android:textSize attribute. The following is a step by step process.
Step 1
Open Android Studio and create an Android Project as shown in the following screenshot.
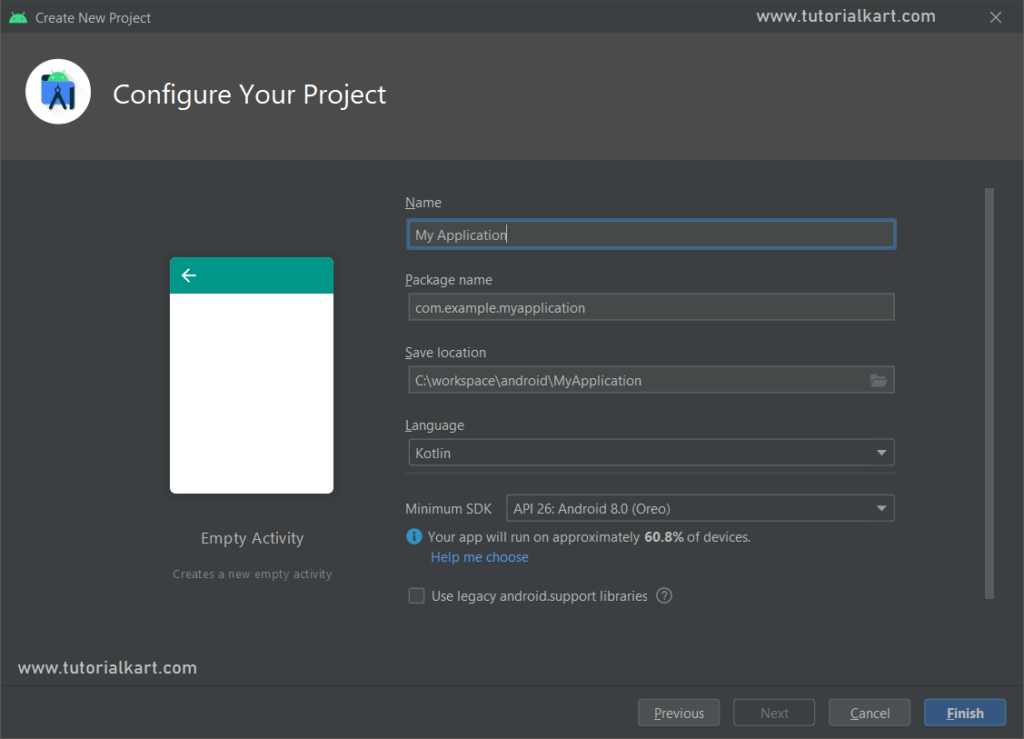
Step 2
Create Button widget in activity_main.xml. Set the text size of Button widget using android:textSize attribute. In the following layout file, we have set the button text size to 24sp.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button"
android:textSize="24sp"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
We may keep the MainActivity.kt to the default code.
MainActivity.kt
package com.example.myapplication
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
}
}
Step 3
Run this Android Application, and we would get the following output in the screen.
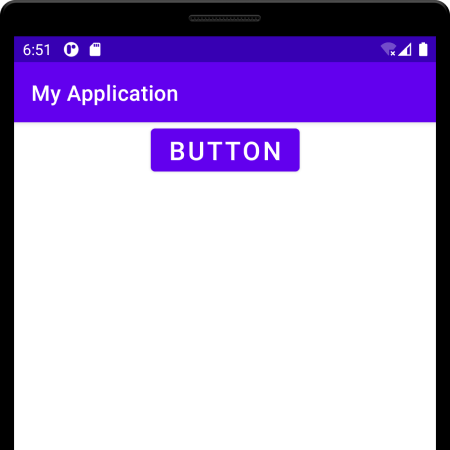
Set Android Button Text Size Programmatically
In this example project, we will change the Button text size programmatically in MainActivity.kt program using Button.setTextSize(unit, size). The following is a step by step process.
Step 1
Open Android Studio and create an Android Project as shown in the following screenshot.
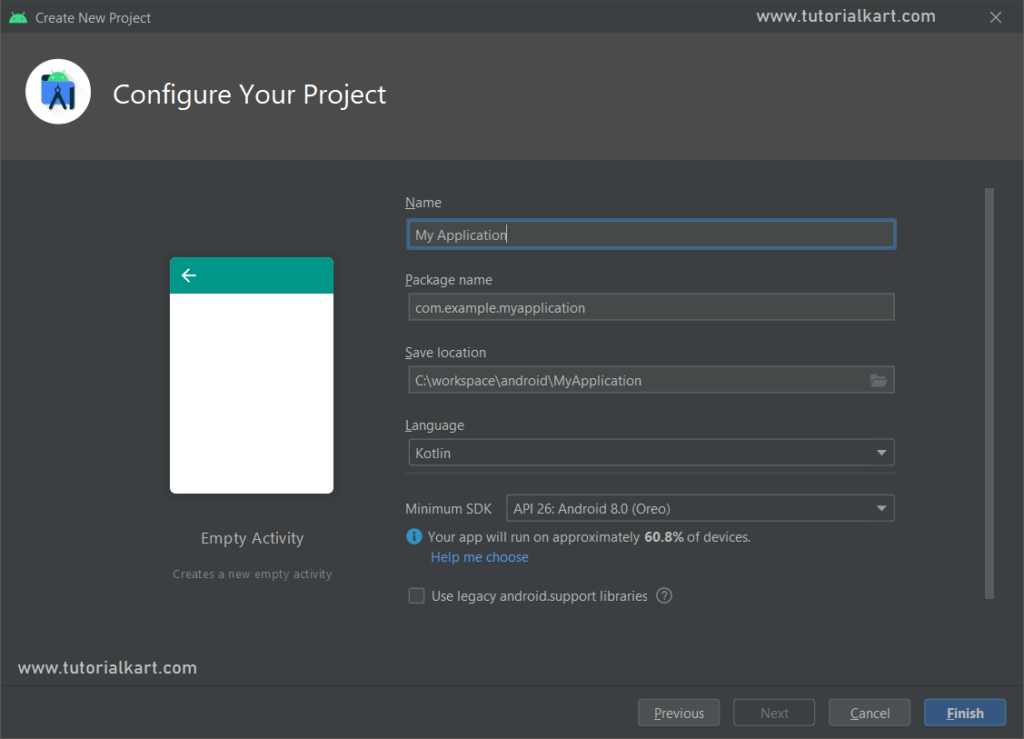
Step 2
Create Button widget in activity_main.xml as shown in the following acitivity_main.xml. Please note that we assigned an id myButton
. We shall use this id to get the reference to this Button in MainActivity.kt program.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/myButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Button"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
In MainActivity.kt program, we will get the reference to the Button using id, and set its text size to 40sp using Button.setTextSize(int unit, float size) method.
MainActivity.kt
package com.example.myapplication
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.util.Size
import android.util.TypedValue
import android.widget.Button
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val button: Button = findViewById(R.id.myButton)
button.setTextSize(TypedValue.COMPLEX_UNIT_SP, 40F)
}
}
Step 3
Run this Android Application, and we would get the following output in the screen.
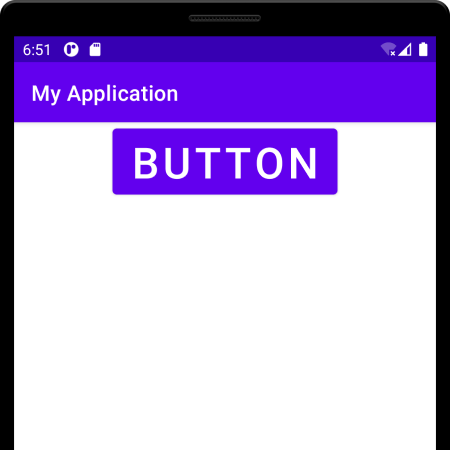
Conclusion
In this Kotlin Android Tutorial, we learned how to change the text size of Button widget in Android using Kotlin language via layout file and programmatically.