Kotlin Android – Remove Shadow of Floating Action Button
To remove shadow of Floating Action Button in Kotlin Android, set the elevation attribute (in layout file) to 0dp or set compatElevation parameter (in Kotlin program) of FAB with floating point value of 0.0f.
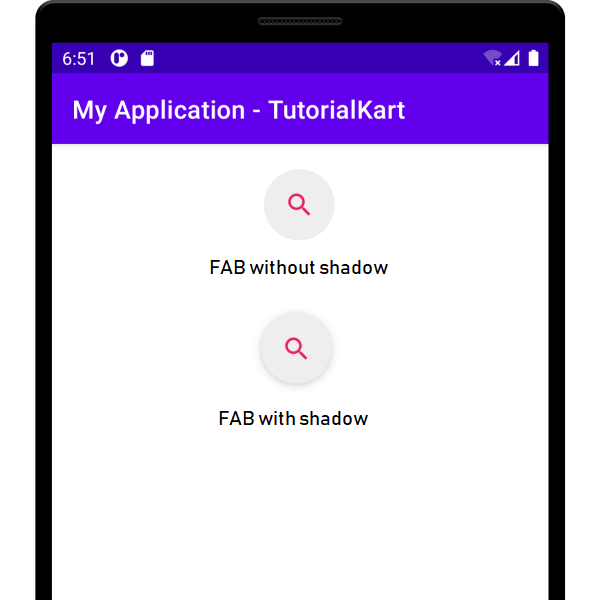
To remove the shadow of Floating Action Button in layout file, set the elevation attribute with zero value as shown in the following code snippet.
xmlns:app="http://schemas.android.com/apk/res-auto" <com.google.android.material.floatingactionbutton.FloatingActionButton ... app:elevation="0dp" />
Please note that the elevation attribute is referenced from xmlns:app=”http://schemas.android.com/apk/res-auto” namespace. Hence, we used app:elevation in the XML.
To remove the shadow of Floating Action Button dynamically or programmatically in Kotlin activity file, set compatElevation parameter of the FAB with 0.0F.
val fab = findViewById<FloatingActionButton>(R.id.floatingActionButton) fab.compatElevation = 0.0f
Example – Remove Shadow of FAB via Layout File
Create an Android Application with Empty Activity and modify the activity_main.xml with the following code.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent"> <com.google.android.material.floatingactionbutton.FloatingActionButton android:id="@+id/floatingActionButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:clickable="true" app:backgroundTint="#EEE" app:elevation="0dp" app:tint="#E91E63" android:layout_marginTop="20dp" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.498" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:srcCompat="?android:attr/actionModeWebSearchDrawable" /> </androidx.constraintlayout.widget.ConstraintLayout>
Keep the MainActivity.kt unchanged.
Run this Android Application, and we would get the output as shown in the following screenshot, with the shadow removed for Floating Action Button (FAB).
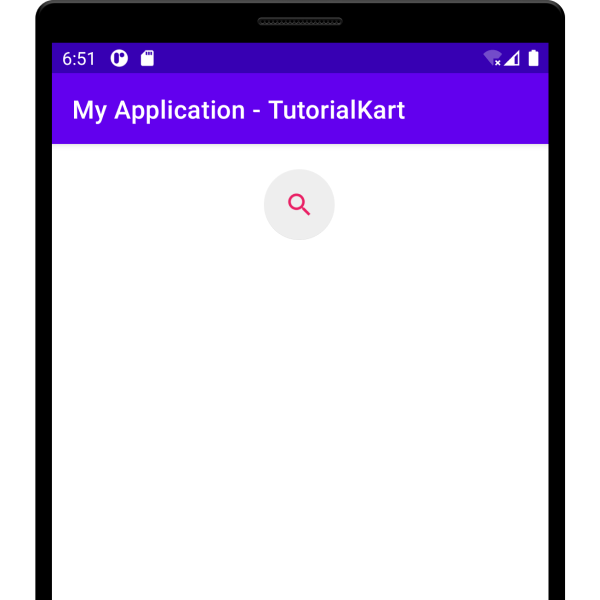
Example – Change Icon Color of FAB Programmatically
Create an Android Application with Empty Activity and modify the activity_main.xml and MainActivity.kt with the following code.
In this example, we will remove the shadow of FAB programmatically in MainActivity.kt file.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent"> <com.google.android.material.floatingactionbutton.FloatingActionButton android:id="@+id/floatingActionButton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:clickable="true" app:backgroundTint="#EEE" app:tint="#E91E63" android:layout_marginTop="20dp" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.498" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" app:srcCompat="?android:attr/actionModeWebSearchDrawable" /> </androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.kt
package com.example.myapplication import androidx.appcompat.app.AppCompatActivity import android.os.Bundle import com.google.android.material.floatingactionbutton.FloatingActionButton class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val fab = findViewById<FloatingActionButton>(R.id.floatingActionButton) fab.compatElevation = 0.0f } }
Run this Android Application, and we would get the output as shown in the following screenshot, with the shadow removed for Floating Action Button (FAB).
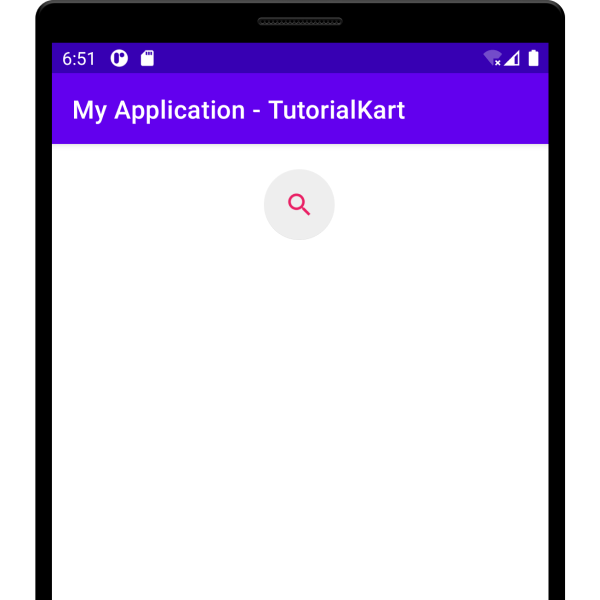
Conclusion
In this Kotlin Android Tutorial, we learned how to remove shadow for Floating Action Button (FAB) via layout file or programmatically in Kotlin Activity file, with examples.