Android Jetpack Compose – AlertDialog
Android Jetpack AlertDialog composable is used to display an alert dialog with actionable items.
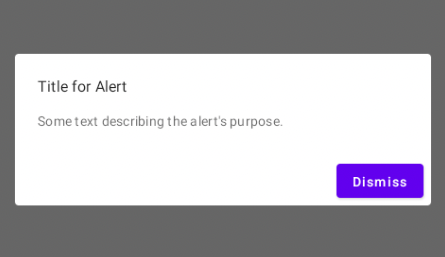
AlertDialog contains title, text, and buttons, all of which are optional.
Examples
Show Alert Dialog on Button Click
In this example, we shall display an AlertDialog, when user clicks on Show Alert
button. Inside the alert, we display title, some text to describe the alert, and a button to dismiss the alert.
We have taken a variable openDialog
with default value of false
. If the value of this variable is true
, then the alert dialog is shown to user. We set openDialog
to true when the user clicks on the button Show Alert
. And inside the alert, we have a button Dismiss
. When user clicks on Dismiss button, we set openDialog
to false
and the AlertDialog disappears.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material.AlertDialog
import androidx.compose.material.Button
import androidx.compose.material.Text
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(modifier = Modifier.padding(20.dp)) {
val openDialog = remember { mutableStateOf(false) }
if (openDialog.value) {
AlertDialog(
onDismissRequest = {
openDialog.value = false
},
title = {
Text(text = "Title for Alert")
},
text = {
Text(
"Some text describing the alert's purpose."
)
},
buttons = {
Row(
modifier = Modifier.padding(all = 8.dp).fillMaxWidth(),
horizontalArrangement = Arrangement.End
) {
Button(
onClick = { openDialog.value = false }
) {
Text("Dismiss")
}
}
}
)
}
Text(
"This is an example for Alert Dialog.",
fontSize = 20.sp,
modifier = Modifier.padding(vertical = 20.dp)
)
Button(onClick = {
openDialog.value = true
}) {
Text("Show Alert")
}
}
}
}
}
}
Screenshot
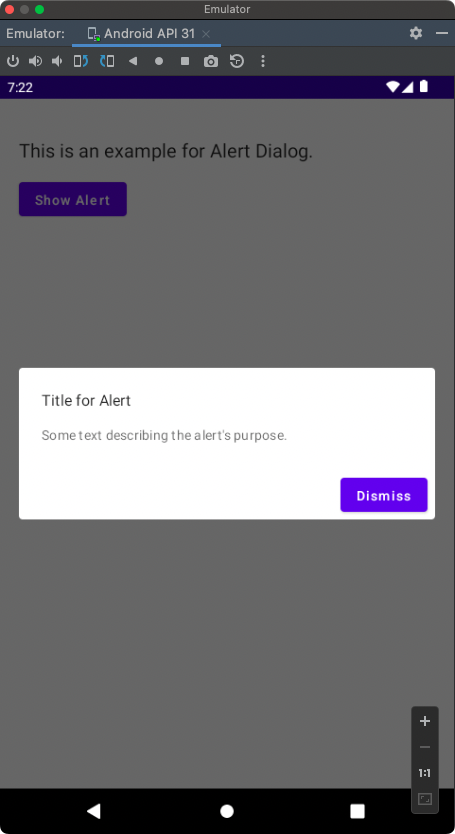
Conclusion
In this Android Jetpack Compose Tutorial, we learned what AlertDialog composable is, and how to set properties like title, text, and buttons for AlertDialog, with the help of examples.