Android Compose – Adjust Image Brightness
In this tutorial, we will learn how to adjust the brightness of an image displayed in Image composable in Android Compose.
Image with original brightness
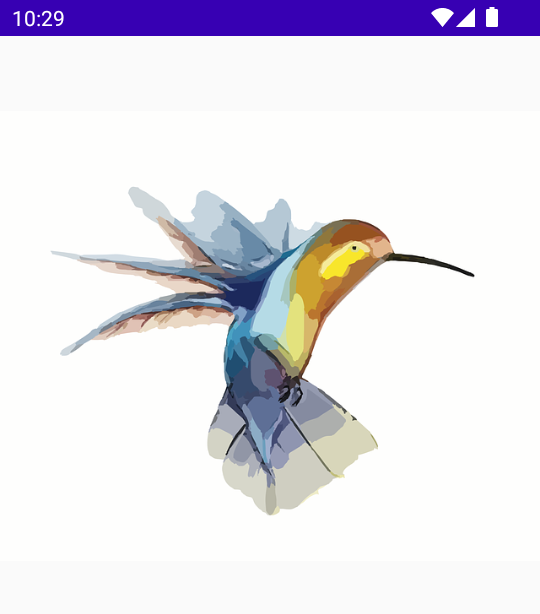
Image with an increased brightness to 100f
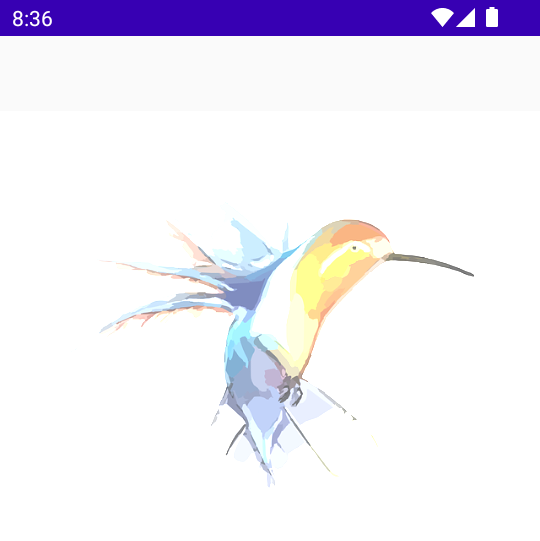
Image with a decreased brightness to -50f
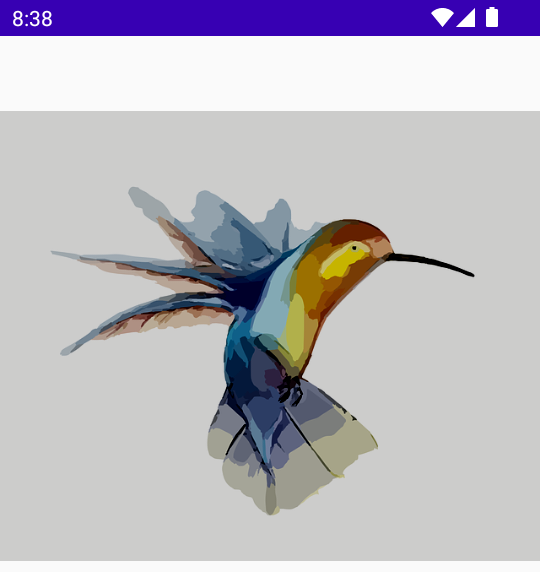
To adjust the brightness of an image in an Image composable in Android Jetpack Compose, use colorFilter parameter of Image(). Set the color matrix with values of required brightness.
val brightness = 100f
val colorMatrix = floatArrayOf(
1f, 0f, 0f, 0f, brightness,
0f, 1f, 0f, 0f, brightness,
0f, 0f, 1f, 0f, brightness,
0f, 0f, 0f, 1f, 0f
)
Image(
painter = painterResource(id = R.drawable.hummingbird),
contentDescription = null,
colorFilter = ColorFilter.colorMatrix(ColorMatrix(colorMatrix))
)
The range of brightness can be varied from -255f
to 255f
, and 0f
is the default value. Rest of the values in the matrix are default values for other parameters in the color matrix.
Refer to color matrix tutorial for more detailed explanation.
Examples (2)
1 Increase brightness of Image to 100f
In this example, we have Compose Activity with an Image composable. We increase the brightness of the image from a default value of 0f to a brightness of 100f.
Screenshot – Image with original brightness
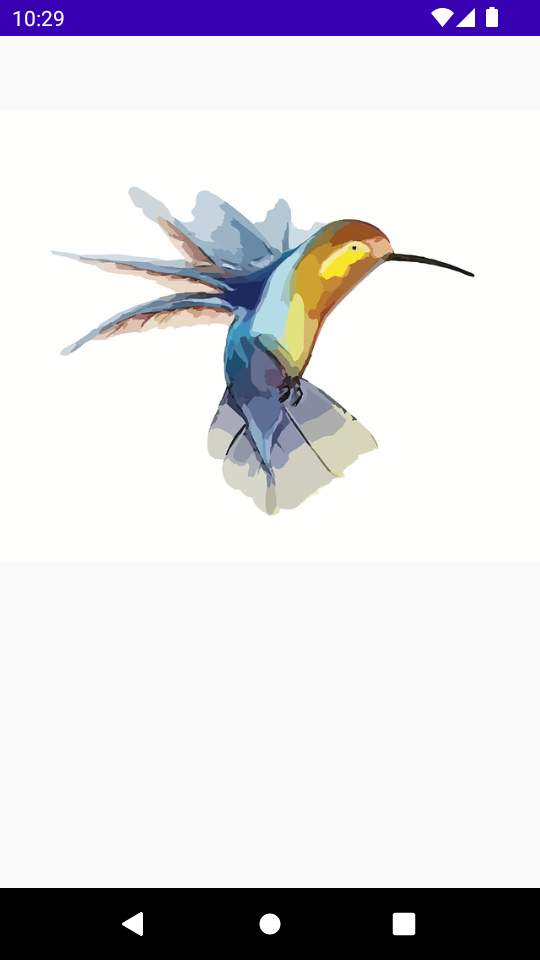
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.ColorFilter
import androidx.compose.ui.graphics.ColorMatrix
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
val brightness = 100f
val colorMatrix = floatArrayOf(
0f, 0f, 0f, 0f, brightness,
0f, 0f, 0f, 0f, brightness,
0f, 0f, 0f, 0f, brightness,
0f, 0f, 0f, 1f, 0f
)
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier.fillMaxWidth(), content = {
Spacer(modifier = Modifier.height(50.dp))
Image(
painter = painterResource(id = R.drawable.hummingbird),
contentDescription = null,
colorFilter = ColorFilter.colorMatrix(ColorMatrix(colorMatrix)),
)
}
)
}
}
}
}
Screenshot – Image with a brightness of 100f
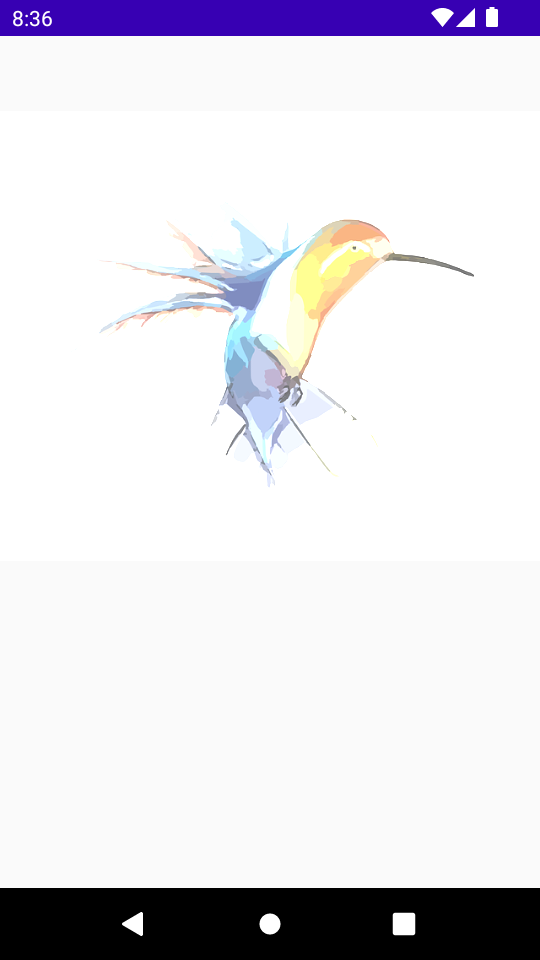
Project Download
The complete Android Studio Project in the above example is available for download at the following link. Download the ZIP file, uncompress it, and open with Android Studio.
2 Decrease brightness of Image to -50f
In the following Image composable, we have decreased the brightness of the image to -50f.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.ColorFilter
import androidx.compose.ui.graphics.ColorMatrix
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
val brightness = -50f
val colorMatrix = floatArrayOf(
1f, 0f, 0f, 0f, brightness,
0f, 1f, 0f, 0f, brightness,
0f, 0f, 1f, 0f, brightness,
0f, 0f, 0f, 1f, 0f
)
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier.fillMaxWidth(), content = {
Spacer(modifier = Modifier.height(50.dp))
Image(
painter = painterResource(id = R.drawable.hummingbird),
contentDescription = null,
colorFilter = ColorFilter.colorMatrix(ColorMatrix(colorMatrix)),
)
}
)
}
}
}
}
Screenshot
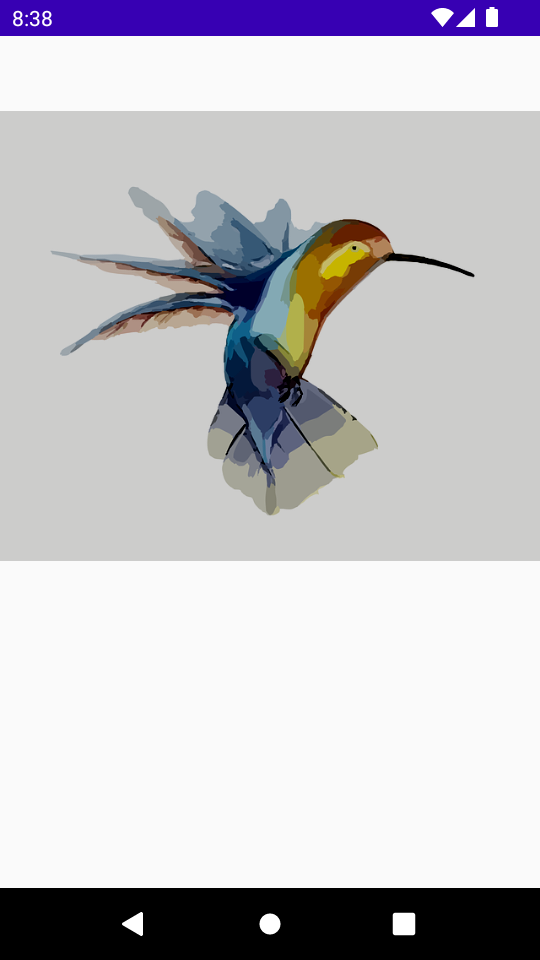
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to adjust the brightness of an Image composable by applying a filter.