Spacer Composable
Spacer component is used to display an empty space. Width and (or) height can be set for Spacer using Modifier object.
The definition of Spacer is
@Composable
fun Spacer(modifier: Modifier) {
Layout({}, modifier) { _, constraints ->
with(constraints) {
val width = if (hasFixedWidth) maxWidth else 0
val height = if (hasFixedHeight) maxHeight else 0
layout(width, height) {}
}
}
}
Spacer composable function accepts Modifier object as parameter, through which we may set the Spacer’s width or (and) height.
Example
In this example, we will display two Rows, and between them we place a Spacer with a height of 10dp.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material.Scaffold
import androidx.compose.material.Text
import androidx.compose.material.TopAppBar
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Scaffold(
topBar = {
TopAppBar(title = {Text("Spacer Example - TutorialKart")})
}
) {
Column(Modifier.padding(5.dp)) {
Row(
modifier = Modifier
.height(50.dp)
.fillMaxWidth()
.background(Color.Gray)
) {}
Spacer(modifier = Modifier.height(20.dp))
Row(
modifier = Modifier
.height(50.dp)
.fillMaxWidth()
.background(Color.Gray)
) {}
}
}
}
}
}
}
Screenshot
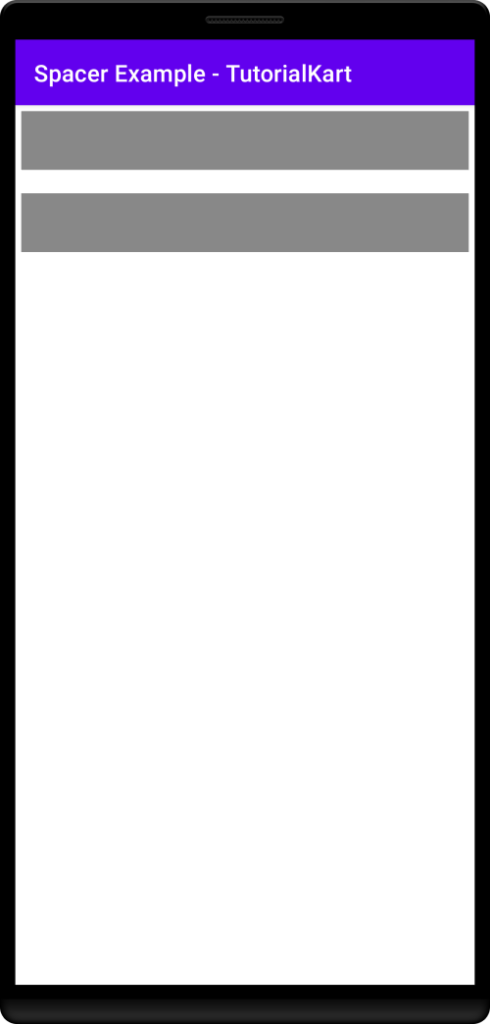
Example – Spacers with Different Height
Now let us change display three rows with two Spacers, the first Spacer with a height of 20dp and the second with a height of 30d.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material.Scaffold
import androidx.compose.material.Text
import androidx.compose.material.TopAppBar
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Scaffold(
topBar = {
TopAppBar(title = {Text("Spacer Example - TutorialKart")})
}
) {
Column(Modifier.padding(5.dp)) {
Row(
modifier = Modifier
.height(50.dp)
.fillMaxWidth()
.background(Color.Gray)
) {}
Spacer(modifier = Modifier.height(20.dp))
Row(
modifier = Modifier
.height(50.dp)
.fillMaxWidth()
.background(Color.Gray)
) {}
Spacer(modifier = Modifier.height(30.dp))
Row(
modifier = Modifier
.height(50.dp)
.fillMaxWidth()
.background(Color.Gray)
) {}
}
}
}
}
}
}
Screenshot
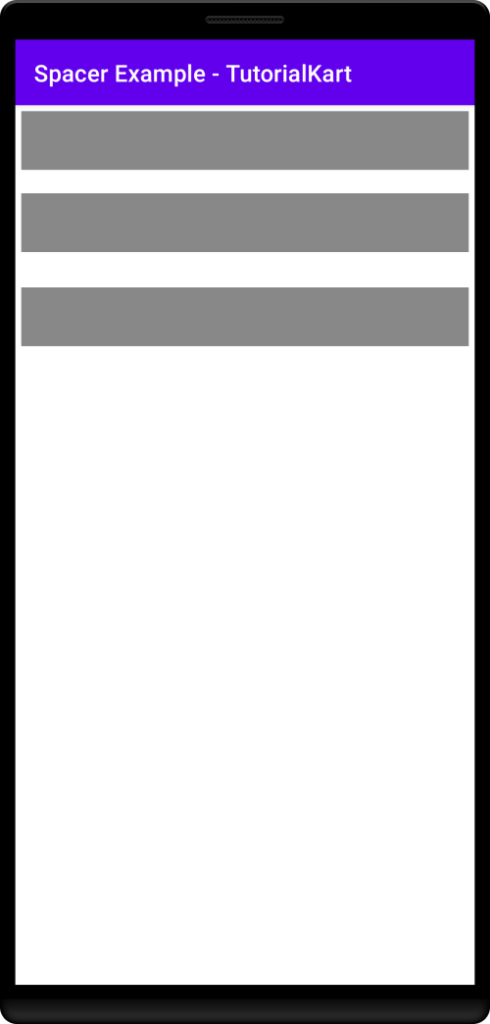
Example – Spacer with Width Set
In this example, we will display two Box composables in a Row, where the boxes are separated by a Spacer with 20dp width.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.background
import androidx.compose.foundation.layout.*
import androidx.compose.material.Scaffold
import androidx.compose.material.Text
import androidx.compose.material.TopAppBar
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Scaffold(
topBar = {
TopAppBar(title = {Text("Spacer Example - TutorialKart")})
}
) {
Row(Modifier.padding(10.dp)){
Box(
modifier = Modifier
.width(50.dp)
.height(50.dp)
.background(Color.Gray)
) {}
Spacer(modifier = Modifier.width(20.dp))
Box(
modifier = Modifier
.width(50.dp)
.height(50.dp)
.background(Color.DarkGray)
) {}
}
}
}
}
}
}
Screenshot
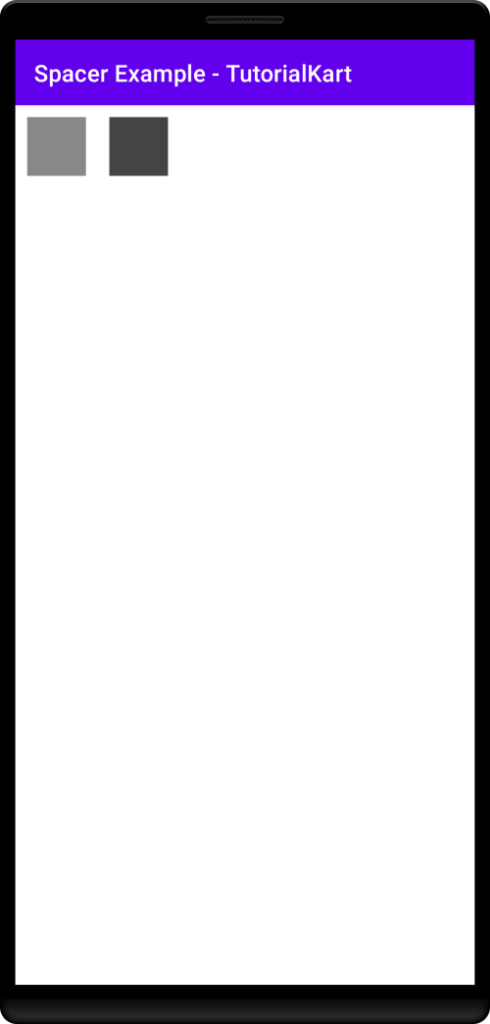
Conclusion
In this Android Jetpack Compose Tutorial, we learned about Spacer in Android Jetpack Compose.