Android Compose – Apply Filter to Image
In this tutorial, we will learn how to apply a filter to an image displayed in Image composable, using color matrix, in Android Compose.
To apply a color filter to an Image, we can use colorFilter parameter of the Image composable.
Please refer color matrix tutorial to learn about the components of the matrix, and how these values in the matrix affect the resulting image.
Examples (4)
1. Display only Red Channel of the Image
In this example, we have apply a filter using color matrix, such that we display only Red channel of the given image in the Image composable.
Screenshot – Original Image
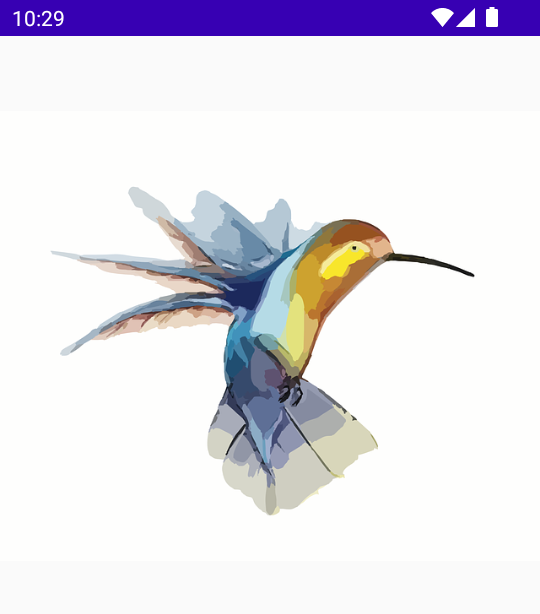
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.ComponentActivity import androidx.activity.compose.setContent import androidx.compose.foundation.Image import androidx.compose.foundation.layout.* import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.graphics.ColorFilter import androidx.compose.ui.graphics.ColorMatrix import androidx.compose.ui.res.painterResource import androidx.compose.ui.unit.dp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { val colorMatrix = floatArrayOf( 1f, 0f, 0f, 0f, 0f, 0f, 0f, 0f, 0f, 0f, 0f, 0f, 0f, 0f, 0f, 0f, 0f, 0f, 1f, 0f ) super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth(), content = { Spacer(modifier = Modifier.height(50.dp)) Image( painter = painterResource(id = R.drawable.hummingbird), contentDescription = null, colorFilter = ColorFilter.colorMatrix(ColorMatrix(colorMatrix)), ) } ) } } } }
Screenshot
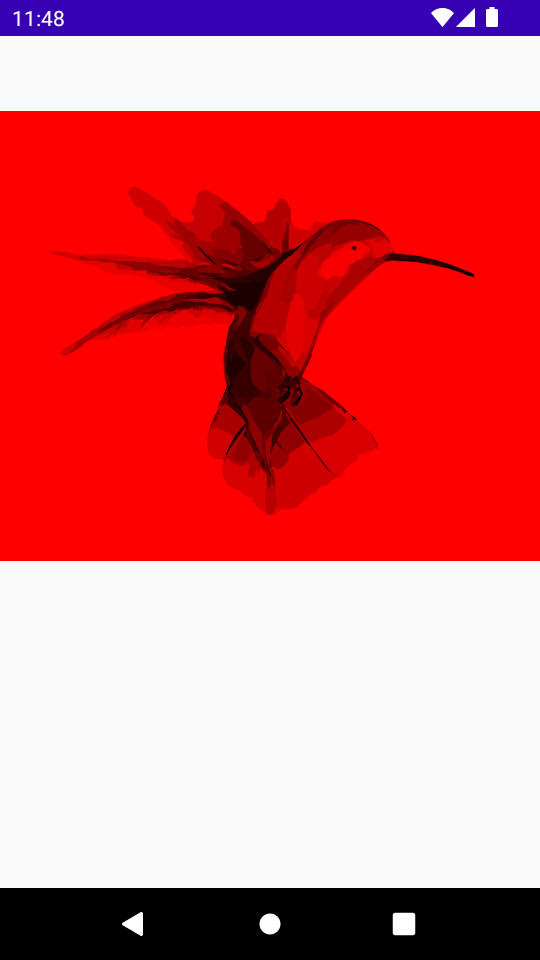
Project Download
The complete Android Studio Project in the above example is available for download at the following link. Download the ZIP file, uncompress it, and open with Android Studio.
2. Display only Blue and Green Channels of the Image
In the following Image composable, we display only blue and green channels of the image.
Color Matrix
Update the color matrix in the first example with the following data.
val colorMatrix = floatArrayOf( 0f, 0f, 0f, 0f, 0f, 0f, 1f, 0f, 0f, 0f, 0f, 0f, 1f, 0f, 0f, 0f, 0f, 0f, 1f, 0f )
Screenshot
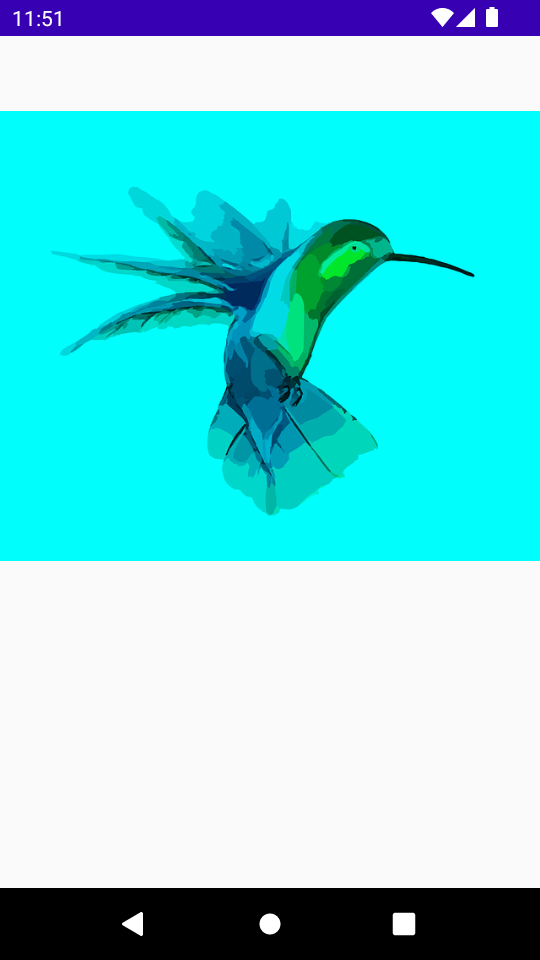
3. Swap Green and Red Channels of the Image
In the following Image composable, we display only blue and green channels of the image.
Color Matrix
Update the color matrix in the first example with the following data.
val colorMatrix = floatArrayOf( 0f, 1f, 0f, 0f, 0f, 1f, 0f, 0f, 0f, 0f, 0f, 0f, 1f, 0f, 0f, 0f, 0f, 0f, 1f, 0f )
Screenshot
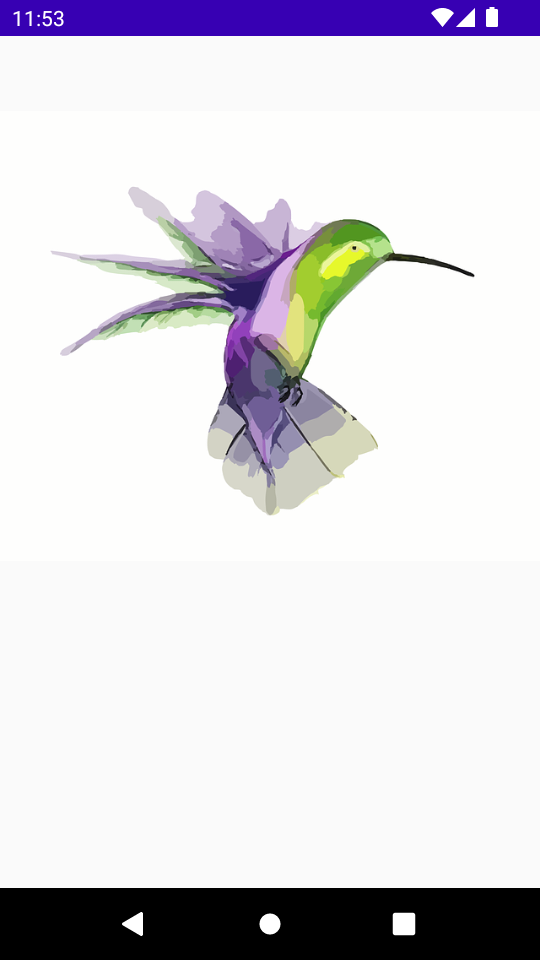
4. Boost Blue Channel of the Image
In the following Image composable, we increase the value of the blue channel by 100f.
Color Matrix
Update the color matrix in the first example with the following data.
val colorMatrix = floatArrayOf( 1f, 0f, 0f, 0f, 100f, 0f, 1f, 0f, 0f, 0f, 0f, 0f, 1f, 0f, 0f, 0f, 0f, 0f, 1f, 0f )
Screenshot
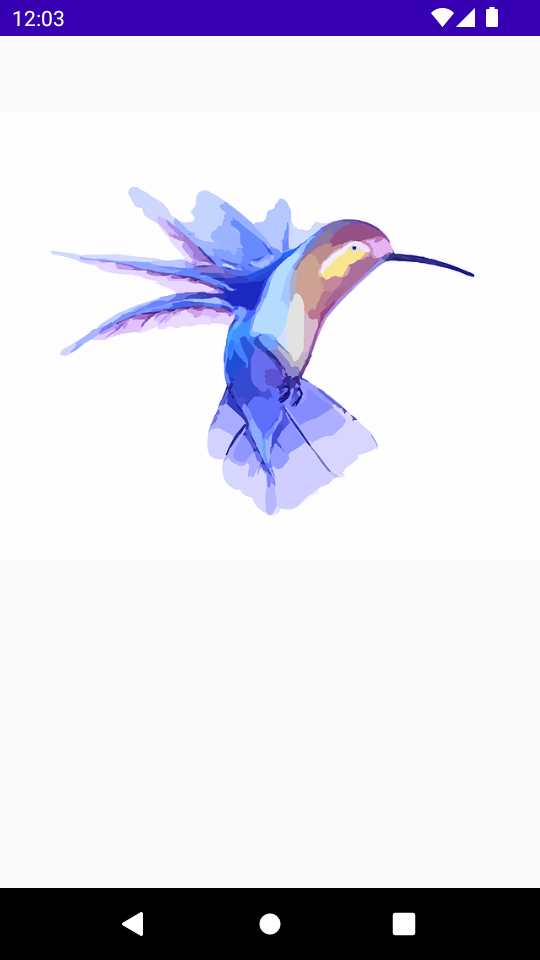
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to apply a filter to an Image using color matrix.