Android Jetpack Compose – Set Height for Button
To set a specific height for Button in Android Jetpack Compose, set modifier
parameter with Modifier companion object and call height() function on this Modifier object. Pass required height in Dp.
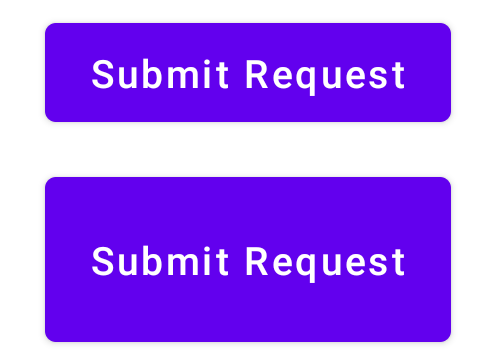
Example
In this example, we shall display two Buttons: the first with default height and the second with a height of 60 dp.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
</>
Copy
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.layout.*
import androidx.compose.material.Button
import androidx.compose.material.Text
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.fillMaxWidth()
.fillMaxHeight()
.padding(20.dp)) {
Button(onClick = { }) {
Text("Submit Request")
}
Spacer(modifier = Modifier.height(20.dp))
Button(onClick = { }, modifier = Modifier.height(60.dp)) {
Text("Submit Request")
}
}
}
}
}
}
Screenshot
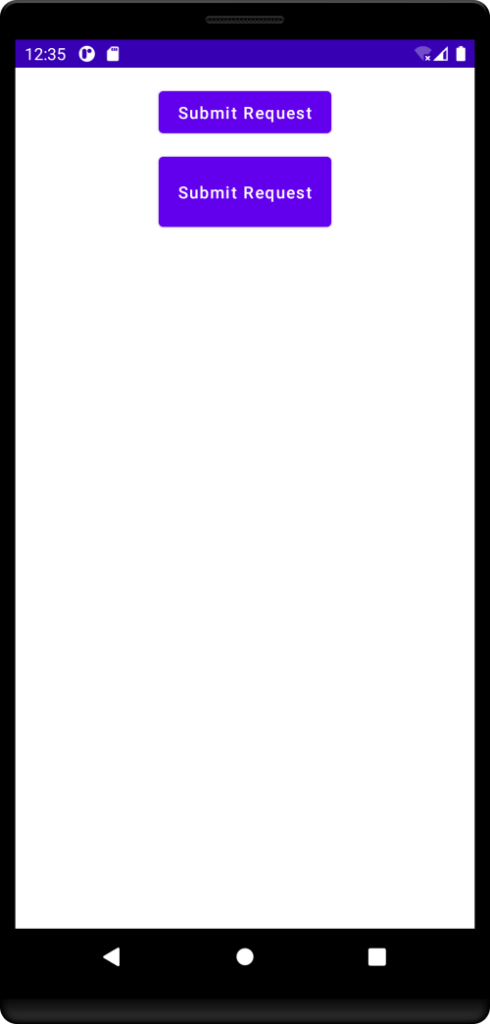
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set the height for Button in Android Jetpack Compose.