Android Compose – Set Border for Image
In this tutorial, we will learn how to set a border of specific border width, border color, and shape for Image composable in Android Compose.
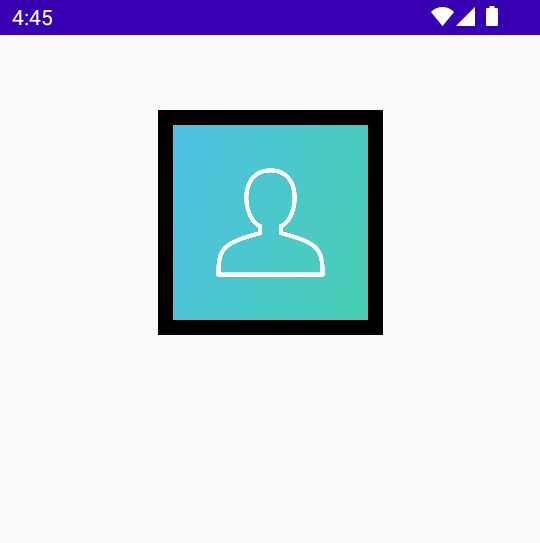
To set a border for an Image composable in Android Jetpack Compose, use modifier parameter of Image. Assign required Modifier object set with border() function. The border() function takes the BorderStroke and Shape as arguments.
Image( painter = painterResource(id = R.drawable.user), contentDescription = null, modifier = Modifier .border( BorderStroke(10.dp, Color.Gray), RectangleShape ) )
Example
In this example, we have Compose Activity with an Image composable. We display the image in circular shape. And we set the image border with a border stroke width of 5.dp, border stroke color or Gray, and border shape of CircleShape.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.ComponentActivity import androidx.activity.compose.setContent import androidx.compose.foundation.BorderStroke import androidx.compose.foundation.Image import androidx.compose.foundation.border import androidx.compose.foundation.layout.* import androidx.compose.foundation.shape.CircleShape import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.draw.clip import androidx.compose.ui.graphics.Color import androidx.compose.ui.res.painterResource import androidx.compose.ui.unit.dp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth(), content = { Spacer(modifier = Modifier.height(50.dp)) Image( painter = painterResource(id = R.drawable.user), contentDescription = null, modifier = Modifier .size(150.dp) .border( BorderStroke(5.dp, Color.Gray), CircleShape ) .clip(CircleShape), ) } ) } } } }
Screenshot
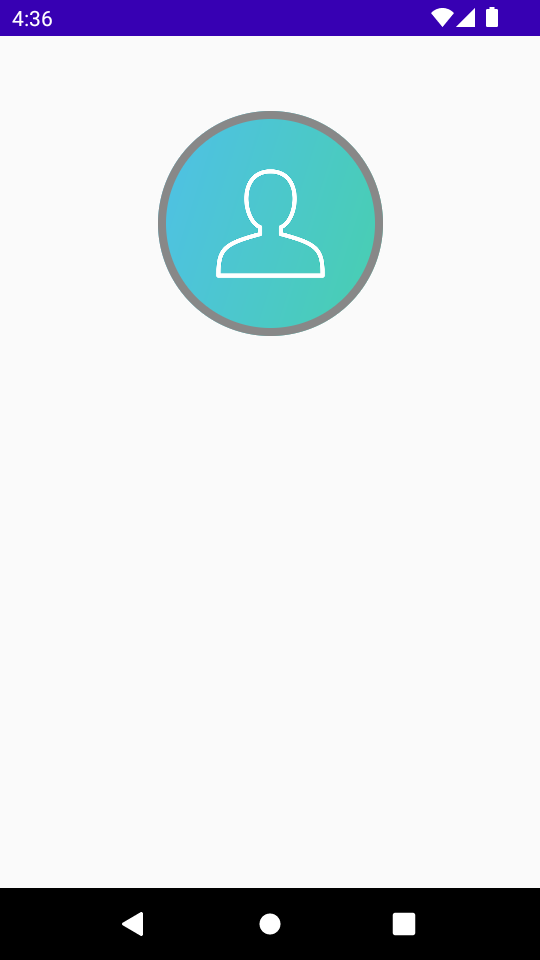
Project Download
The complete Android Studio Project in the above example is available for download at the following link. Download the ZIP file, uncompress it, and open with Android Studio.
Rectangle Shape Border for Image
In the following code for Image composable, we display the image in a rectangle shape, and set its border to rectangle shape. The border is of black color and has a stroke width of 10.dp.
Replace the Image composable in the first example, with the following code.
Code for Image Composable
Image( painter = painterResource(id = R.drawable.user), contentDescription = null, modifier = Modifier .size(150.dp) .border( BorderStroke(10.dp, Color.Gray), RectangleShape ) )
Screenshot
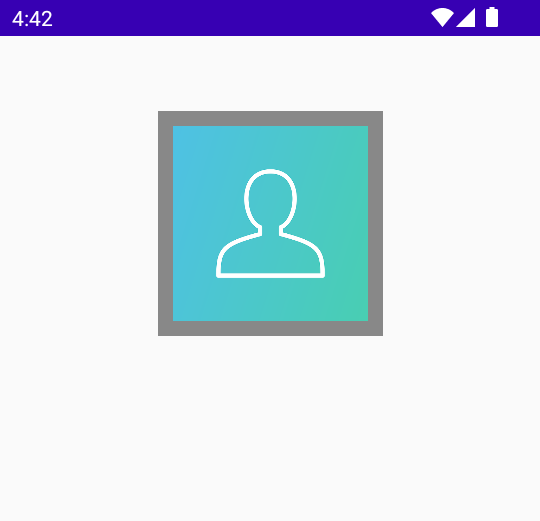
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set border for an Image composable.