Android Compose – Button Border
In this tutorial, we will learn how to set a border for a Button in Android Compose. We can set border stroke color, and width.
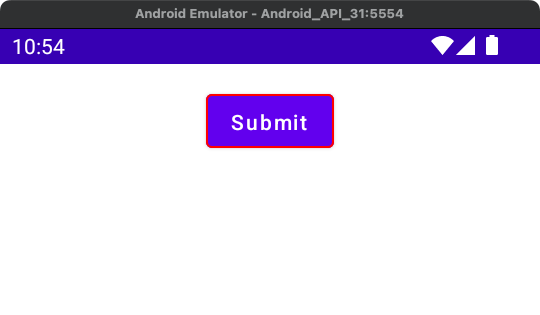
To set border for the Button in Android Jetpack Compose, set border parameter with the required BroderStroke value.
Button(border = BorderStroke(1.dp, Color.Red),)
Example
In this example, we have UI with a Button. The button has the text Submit
. And we set border for this Button with a border stroke width of 1.dp
and a border stroke color of Color.Red
.
Create a Project in Android Studio with empty compose activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.ComponentActivity import androidx.activity.compose.setContent import androidx.compose.foundation.BorderStroke import androidx.compose.foundation.layout.* import androidx.compose.material.Button import androidx.compose.material.Text import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.graphics.Color import androidx.compose.ui.platform.LocalContext import androidx.compose.ui.unit.dp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier .padding(20.dp) .fillMaxWidth()) { val context = LocalContext.current Button( border = BorderStroke(1.dp, Color.Red), onClick = {} ) { Text("Submit") } } } } } }
Screenshot
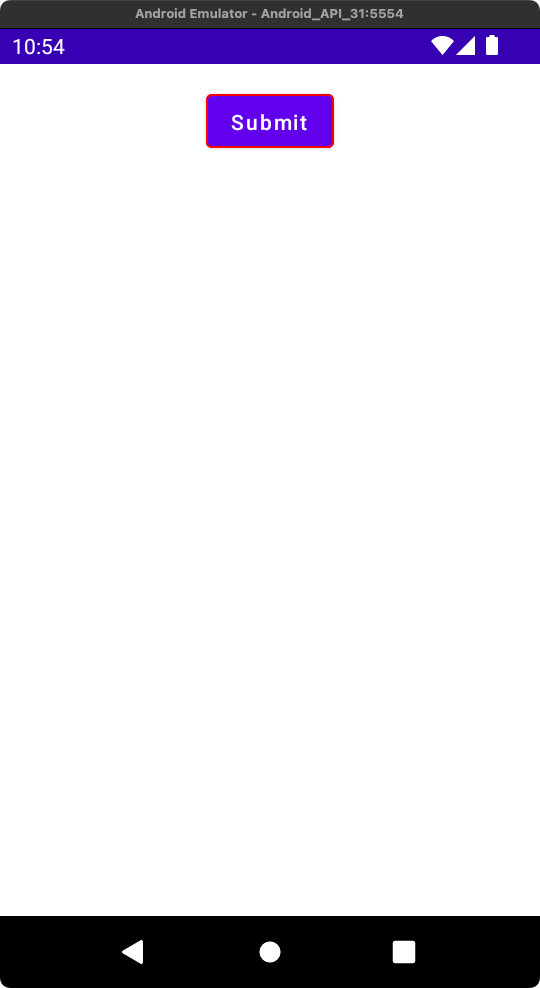
Compose Button with Border of stroke width of 4 dp and Gray color
Now, let us change the border width to 4.dp
and border color to Color.Gray
.
Code for Button
Button( border = BorderStroke(2.dp, Color.Gray), onClick = {} ) { Text("Submit") }
Screenshot
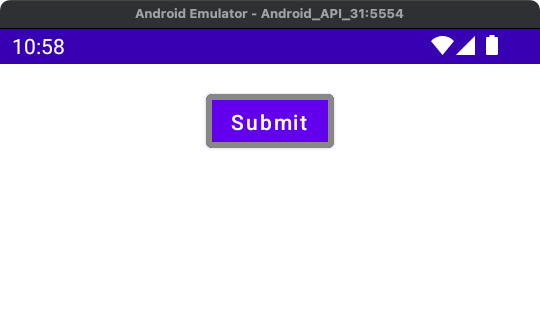
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set border for a Button with specific border stroke color and width using border parameter.