Android Compose – Set Label for TextField
To set label for TextField in Android Compose, assign required value as Text composable for label
parameter of TextField. label
parameter of TextField accepts Composable only.
The following code snippet shows how to set label text for TextField with Enter text
.
var username by remember { mutableStateOf("") }
TextField(
value = username,
onValueChange = { username = it },
label = { Text("Enter text") }
)
Please note that we are not assigning a string value directly to label, but assigning a Text composable prepared with the label text.
Example
In this example, let us create a TextField and set its label text with Enter text
.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.fillMaxWidth
import androidx.compose.material.Text
import androidx.compose.material.TextField
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.runtime.remember
import androidx.compose.runtime.getValue
import androidx.compose.runtime.setValue
import androidx.compose.runtime.mutableStateOf
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier.fillMaxWidth()
) {
var username by remember { mutableStateOf("") }
TextField(
value = username,
onValueChange = { username = it },
label = { Text("Enter text") }
)
}
}
}
}
}
Screenshot
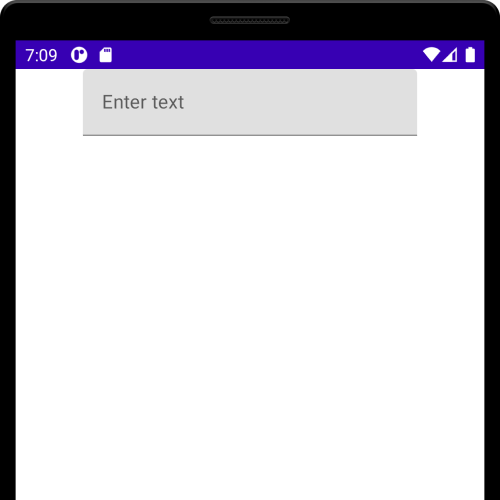
When user clicks on the TextField, the label moves to the top left corner.
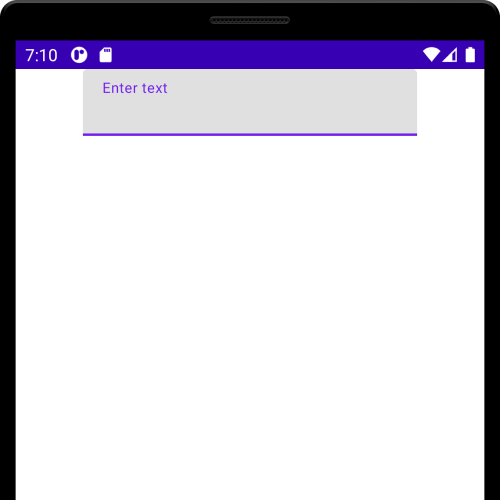
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set the label text for TextField, using label
parameter.