Android Jetpack Compose – Set Image as Background for Card
To set an image as the background for Card in Android Jetpack Compose, specify the first element in the Card with Image composable, and the next with the content you would like to display on the card.
When we place two composables in a Card, there is no guidance on their arrangement. By default, when no guidance is provided for arrangement of composables, they are arranged in stack, which in this case, the Image is displayed, and on top of this the rest of the elements are displayed in UI.
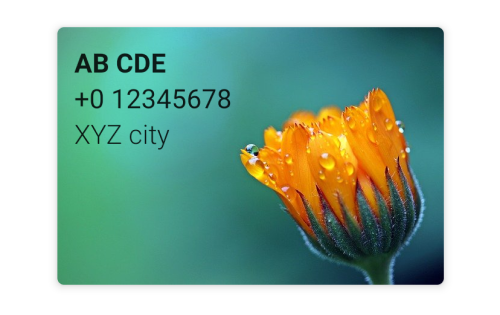
Example
In this example, we shall display a Card composable and set its background with an image from drawable resources.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.*
import androidx.compose.material.Card
import androidx.compose.material.Text
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.text.font.FontWeight
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.fillMaxWidth()
.fillMaxHeight()
.padding(20.dp)) {
Card(
elevation = 4.dp,
) {
Image(painter = painterResource(id = R.drawable.flower), contentDescription = null)
Column(modifier = Modifier.padding(10.dp)) {
Text("AB CDE", fontWeight = FontWeight.W700)
Text("+0 12345678")
Text("XYZ city", fontWeight = FontWeight.W300)
}
}
}
}
}
}
}
Screenshot
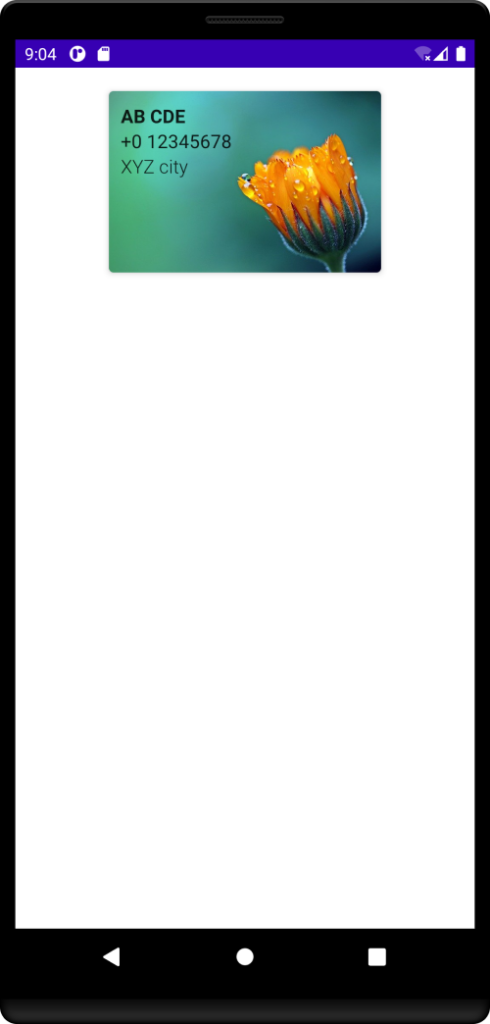
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set an image as background for Card composable in Android Jetpack Compose.