Android Compose – Adjust Image Alpha/Transparency
In this tutorial, we will learn how to adjust the transparency of an image displayed in Image composable in Android Compose.
Image with original alpha
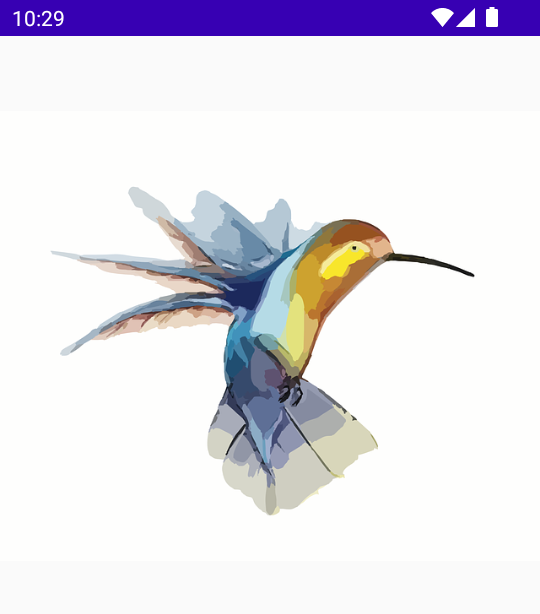
Image with an alpha set to 0.3f – Transparency (70%)
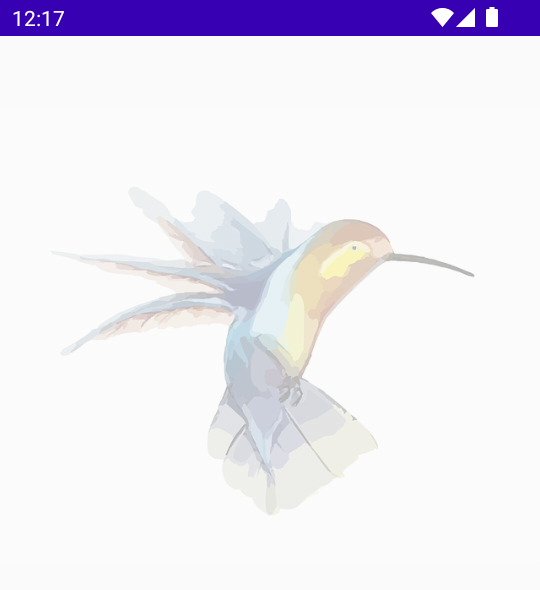
To adjust the transparency of an image displayed in Image composable, take required color matrix with changes made to the alpha channel. And set the colorFilter parameter of the Image composable with the ColorFilter object created from the color matrix.
val alpha = 0.3f val colorMatrix = floatArrayOf( 1f, 0f, 0f, 0f, 0f, 0f, 1f, 0f, 0f, 0f, 0f, 0f, 1f, 0f, 0f, 0f, 0f, 0f, alpha, 0f ) Image( painter = painterResource(id = R.drawable.hummingbird), contentDescription = null, colorFilter = ColorFilter.colorMatrix(ColorMatrix(colorMatrix)) )
The range of alpha can be varied from 0f
to 1f
, and 1f
is the default value.
Refer to color matrix tutorial for more detailed explanation.
Examples
1. Set alpha of Image composable to 0.3f
In this example, we have Compose Activity with an Image composable. We set the alpha of the image from a default value of 1f
to a value of 0.3f
.
Screenshot – Image with original contrast
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.ComponentActivity import androidx.activity.compose.setContent import androidx.compose.foundation.Image import androidx.compose.foundation.layout.* import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.graphics.ColorFilter import androidx.compose.ui.graphics.ColorMatrix import androidx.compose.ui.res.painterResource import androidx.compose.ui.unit.dp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { val alpha = 0.3f val colorMatrix = floatArrayOf( 1f, 0f, 0f, 0f, 0f, 0f, 1f, 0f, 0f, 0f, 0f, 0f, 1f, 0f, 0f, 0f, 0f, 0f, alpha, 0f ) super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth(), content = { Spacer(modifier = Modifier.height(50.dp)) Image( painter = painterResource(id = R.drawable.hummingbird), contentDescription = null, colorFilter = ColorFilter.colorMatrix(ColorMatrix(colorMatrix)), ) } ) } } } }
Screenshot
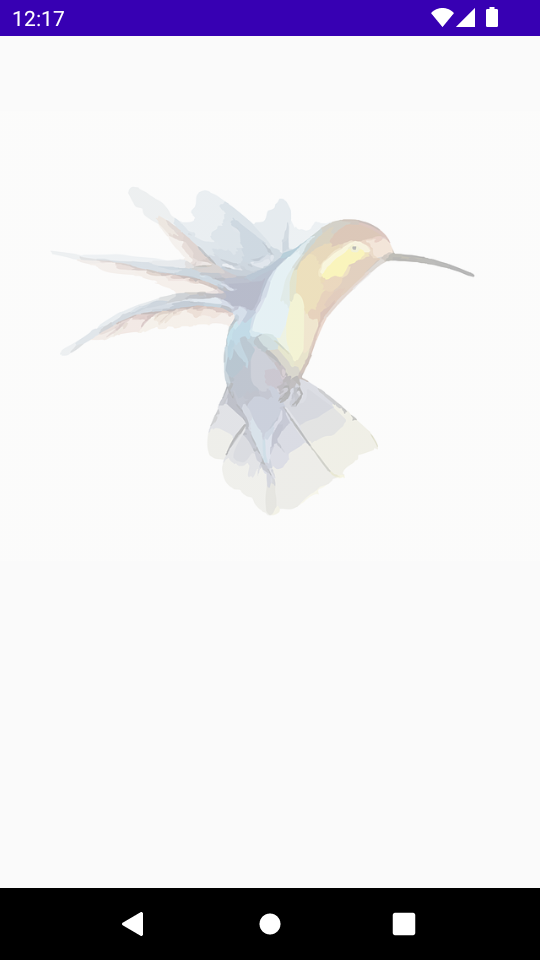
Project Download
The complete Android Studio Project in the above example is available for download at the following link. Download the ZIP file, uncompress it, and open with Android Studio.
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to adjust the transparency of an Image composable by applying a filter.