Android Compose – Read Value Entered in TextField
To read value entered in TextField in Android Compose, declare a variable, and assign this variable the value in the TextField whenever there is a change in the value.
The same process holds for reading value entered in OutlineTextField composable.
The following code snippet shows how to achieve the above solution.
var username by remember { mutableStateOf("") } TextField( value = username, onValueChange = { username = it }, label = { Text("Label") } )
We use remember
for the variable, to prevent recalculating the value for TextField whenever recomposition happens for the TextField during Application state changes.
Example
In this example, let us create a TextField to enable user to enter input. Then we shall read the value using variable text
, and display it in a Text composable below the TextField.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.compose.setContent import androidx.appcompat.app.AppCompatActivity import androidx.compose.foundation.layout.Column import androidx.compose.foundation.layout.fillMaxWidth import androidx.compose.material.OutlinedTextField import androidx.compose.material.Text import androidx.compose.material.TextField import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.runtime.remember import androidx.compose.runtime.getValue import androidx.compose.runtime.setValue import androidx.compose.runtime.mutableStateOf import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth() ) { var username by remember { mutableStateOf("") } TextField( value = username, onValueChange = { username = it }, label = { Text("Label") } ) Text(username) } } } } }
Whenever user changes the value in the TextField, the username
variable is loaded with the current value, through which we can access the value in TextField.
Screenshot
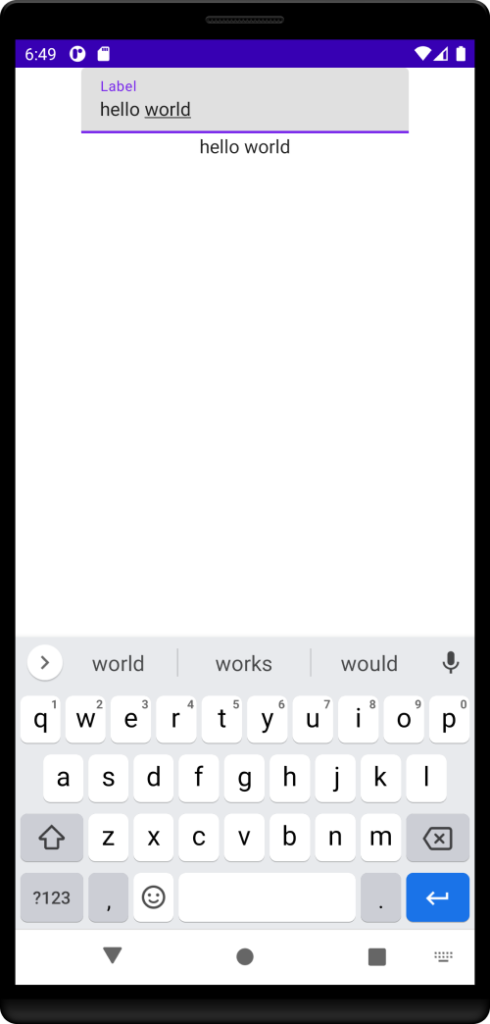
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to read the value entered in TextField, using onValueChange parameter.