Android Compose – Gradient Border for Image
In our previous tutorial, we learned how to set a border for an Image composable.
In this tutorial, we will learn how to set a gradient border for Image composable in Android Compose.
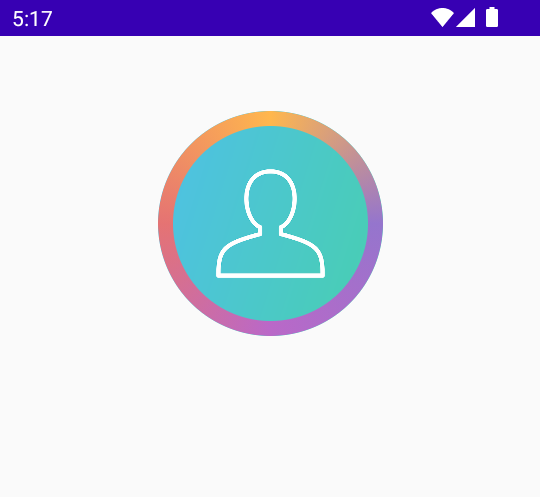
To set a gradient border for an Image composable in Android Jetpack Compose, use modifier parameter of Image. As shown in the following code snippet, set required border using Modifier.border().
BorderStroke(width, brush) can be used to specify a Brush object with required list of colors for gradient.
Image( painter = painterResource(id = R.drawable.user), contentDescription = null, modifier = Modifier .size(150.dp) .clip(CircleShape) .border( BorderStroke( 10.dp, Brush.sweepGradient( listOf( Color(0xFF9575CD), Color(0xFFBA68C8), Color(0xFFE57373), Color(0xFFFFB74D), Color(0xFF9575CD), ) )), CircleShape ) )
Types of gradients
Along with sweep gradient of colors, we can also set border with linear gradient, vertical gradient, horizontal gradient, etc.
Examples
1. Image with Sweep Gradient Border
In this example, we have Compose Activity with an Image composable. We display the image in circular shape, and set the image border with a sweep gradient.
Pass the list of colors as argument to the Brush.sweepGradient() function, as shown in the following program.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.ComponentActivity import androidx.activity.compose.setContent import androidx.compose.foundation.BorderStroke import androidx.compose.foundation.Image import androidx.compose.foundation.border import androidx.compose.foundation.layout.* import androidx.compose.foundation.shape.CircleShape import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.draw.clip import androidx.compose.ui.graphics.Brush import androidx.compose.ui.graphics.Color import androidx.compose.ui.res.painterResource import androidx.compose.ui.unit.dp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth(), content = { Spacer(modifier = Modifier.height(50.dp)) Image( painter = painterResource(id = R.drawable.user), contentDescription = null, modifier = Modifier .size(150.dp) .clip(CircleShape) .border( BorderStroke( 10.dp, Brush.sweepGradient( listOf( Color(0xFF9575CD), Color(0xFFBA68C8), Color(0xFFE57373), Color(0xFFFFB74D), Color(0xFF9575CD), ) )), CircleShape ) ) } ) } } } }
Please note that for sweep gradient, we have specified a same color for first and last items in the list.
Screenshot
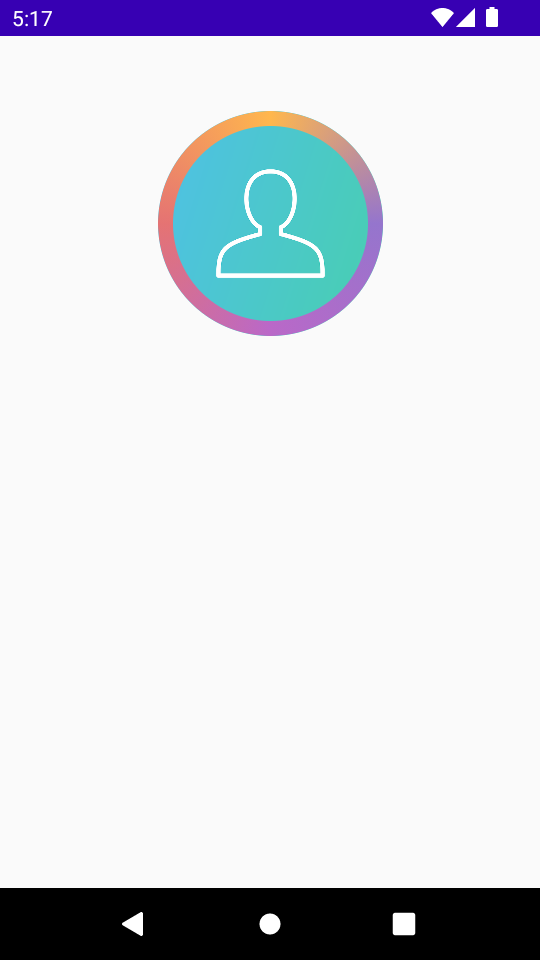
Project Download
The complete Android Studio Project in the above example is available for download at the following link. Download the ZIP file, uncompress it, and open with Android Studio.
2. Image with Horizontal Gradient Border
In the following example, we display an Image with horizontal gradient border.
For horizontal gradient border, the given list of colors are used to color the border from left to right horizontally.
Replace the Image composable in the first example, with the following code.
Code for Image Composable
Image( painter = painterResource(id = R.drawable.user), contentDescription = null, modifier = Modifier .size(150.dp) .clip(CircleShape) .border( BorderStroke( 10.dp, Brush.horizontalGradient( listOf( Color(0xFF9575CD), Color(0xFFBA68C8), Color(0xFFE57373), Color(0xFFFFB74D), Color(0xFF8BC34A), ) )), CircleShape ) )
Screenshot
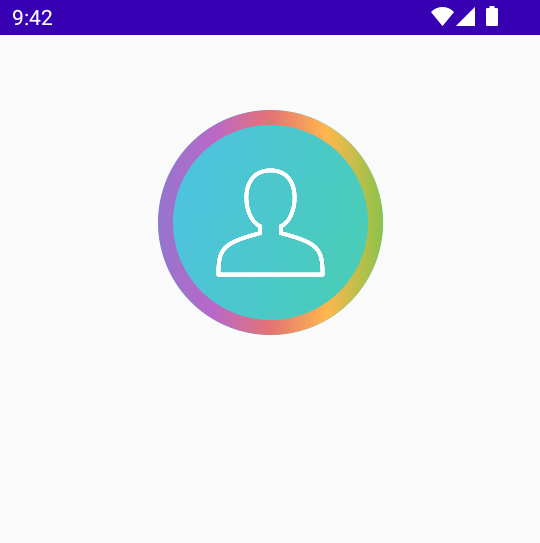
3. Image with Vertical Gradient Border
In the following example, we display an Image with vertical gradient border.
For vertical gradient border, the given list of colors are used to color the border from top to bottom vertically.
Replace the Image composable in the first example, with the following code.
Code for Image Composable
Image( painter = painterResource(id = R.drawable.user), contentDescription = null, modifier = Modifier .size(150.dp) .clip(CircleShape) .border( BorderStroke( 10.dp, Brush.verticalGradient( listOf( Color(0xFF9575CD), Color(0xFFBA68C8), Color(0xFFE57373), Color(0xFFFFB74D), Color(0xFF8BC34A), ) )), CircleShape ) )
Screenshot
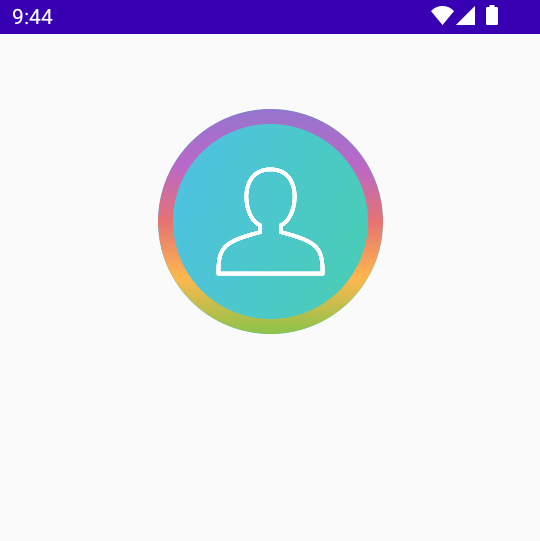
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set gradient border for an Image composable.