Column – Center Align Horizontally
To center align content of Column along horizontal axis in Android Compose, set horizontalAlignment
parameter with the value of Alignment.CenterHorizontally
.
Also, we may fill the maximum width by the Column using Modifier.fillMaxWidth().
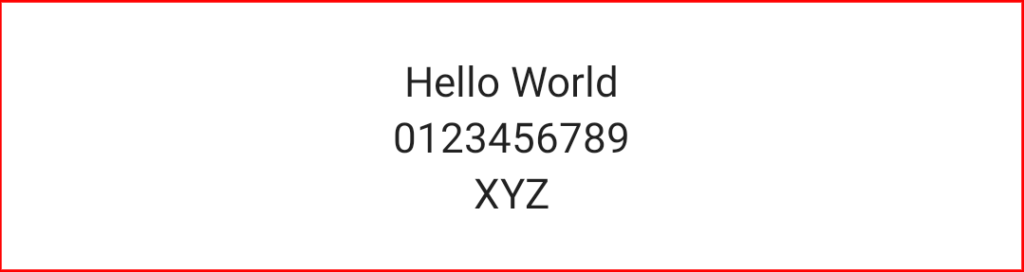
Example
In this example, we shall display a Column with three Text composables as its content. We shall center align the content of Column along its horizontal axis, in its available width.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.border
import androidx.compose.foundation.layout.*
import androidx.compose.material.Text
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.graphics.RectangleShape
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.border(1.dp, Color.Red, RectangleShape)
.fillMaxWidth()
.padding(20.dp)) {
Text("Hello World")
Text("0123456789")
Text("XYZ")
}
}
}
}
}
Please note that the Column is filled in the maximum width available.
Screenshot
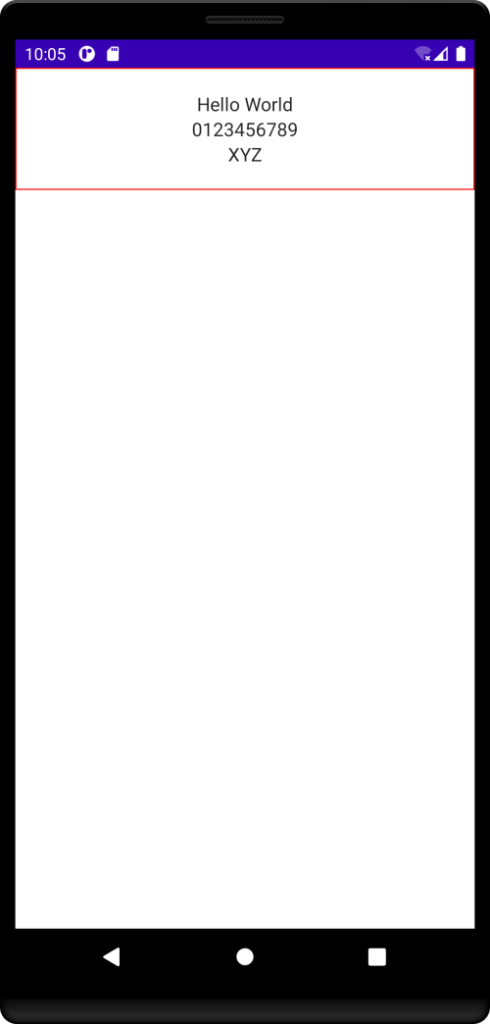
If the Column is not filled in the maximum width available, the width of the Column is set to the maximum width of the content. In the following example, the Column is defined with its default width. No, width is specified explicitly.
MainActivity.kt
package com.example.myapplication
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.appcompat.app.AppCompatActivity
import androidx.compose.foundation.border
import androidx.compose.foundation.layout.*
import androidx.compose.material.Text
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.graphics.RectangleShape
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.border(1.dp, Color.Red, RectangleShape)
.padding(20.dp)) {
Text("Hello World")
Text("0123456789")
Text("XYZ")
}
}
}
}
}
The border for Column is set to red color, so that we can understand boundaries of this Column.
Screenshot
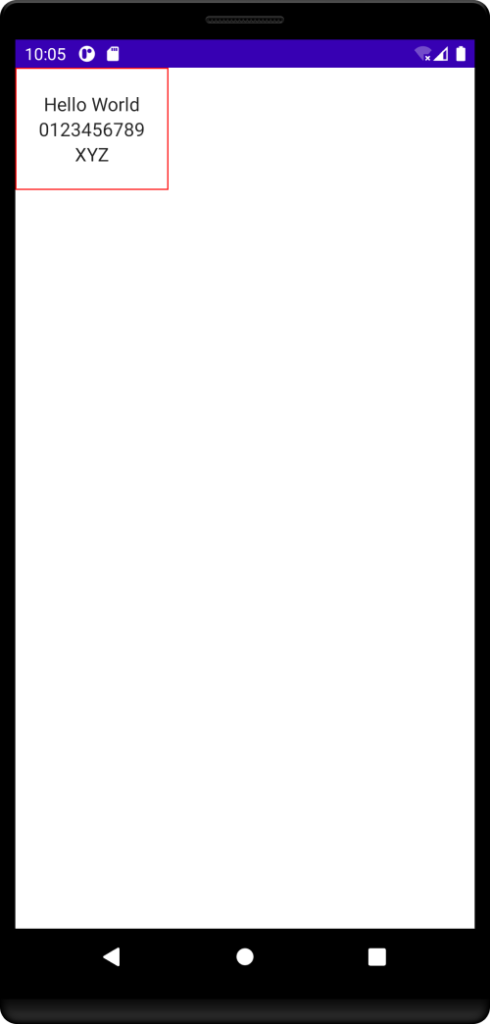
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to center align the content of Column, along horizontal axis, in Android Jetpack Compose.