Android Compose – Scale an Image
In this tutorial, we will learn how to scale an image displayed in Image composable in Android Compose.
To scale an image displayed in Image composable, you can use the contentScale parameter. Set the contentScale parameter with the required scaling value. The scaling value can be any of the following ContentScale value.
- ContentScale.Crop
- ContentScale.FillBounds
- ContentScale.Fit
- ContentScale.FillHeight
- ContentScale.FillWidth
- ContentScale.Inside
- ContentScale.None
Image( painter = painterResource(id = R.drawable.hummingbird), contentDescription = null, contentScale = ContentScale.Crop, )
We shall go through each of the above values in the list with an example.
We shall consider the following image, whose dimensions are 429×640.
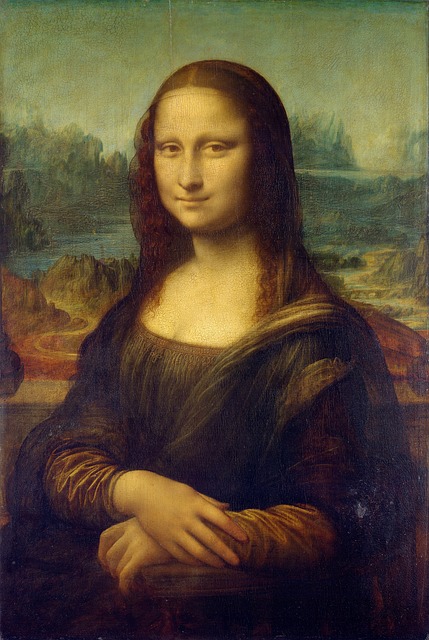
We shall scale this image to different values.
Scale Image – Crop
ContentScale.Crop
value center crops the image into the available space for the Image composable.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.ComponentActivity import androidx.activity.compose.setContent import androidx.compose.foundation.BorderStroke import androidx.compose.foundation.Image import androidx.compose.foundation.border import androidx.compose.foundation.layout.* import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.graphics.Color import androidx.compose.ui.graphics.RectangleShape import androidx.compose.ui.layout.ContentScale import androidx.compose.ui.res.painterResource import androidx.compose.ui.unit.dp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : ComponentActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth(), content = { Spacer(modifier = Modifier.height(50.dp)) Image( painter = painterResource(id = R.drawable.monalisa), contentDescription = null, contentScale = ContentScale.Crop, modifier = Modifier .size(200.dp) .border( BorderStroke(10.dp, Color.Gray), RectangleShape ) ) } ) } } } }
Screenshot
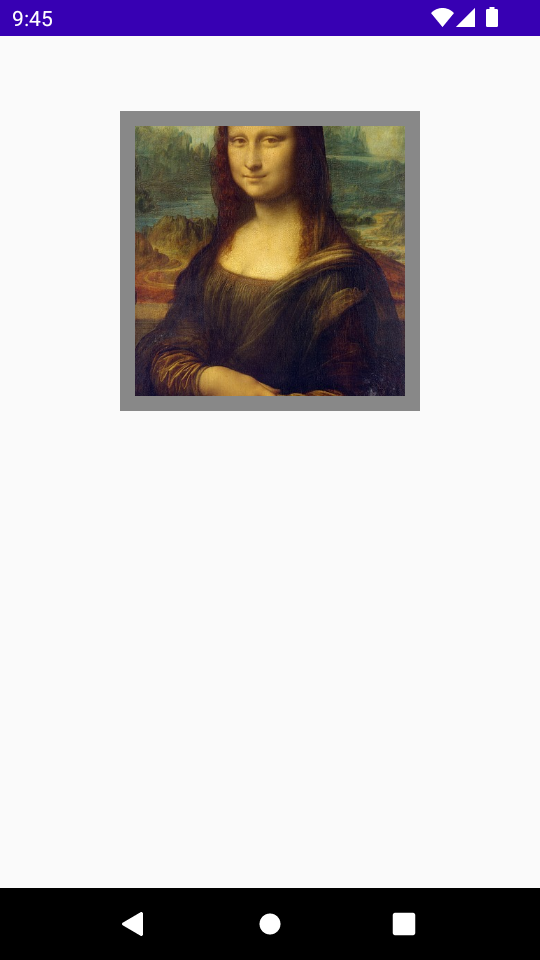
Project Download
The complete Android Studio Project in the above example is available for download at the following link. Download the ZIP file, uncompress it, and open with Android Studio.
Scale Image – Fit
ContentScale.Fit
value scales the image uniformly, keeping the aspect ratio (default).
If image is smaller than the available size, the image is scaled up to fit the bounds.
If image is larger than the available size, the image is scaled down to fit the bounds.
Copy and replace the Image composable code in the MainActivity.kt with the following code.
Code for Image Composable
Image( painter = painterResource(id = R.drawable.monalisa), contentDescription = null, contentScale = ContentScale.Fit, modifier = Modifier .size(200.dp) .border( BorderStroke(10.dp, Color.Gray), RectangleShape ) )
Screenshot
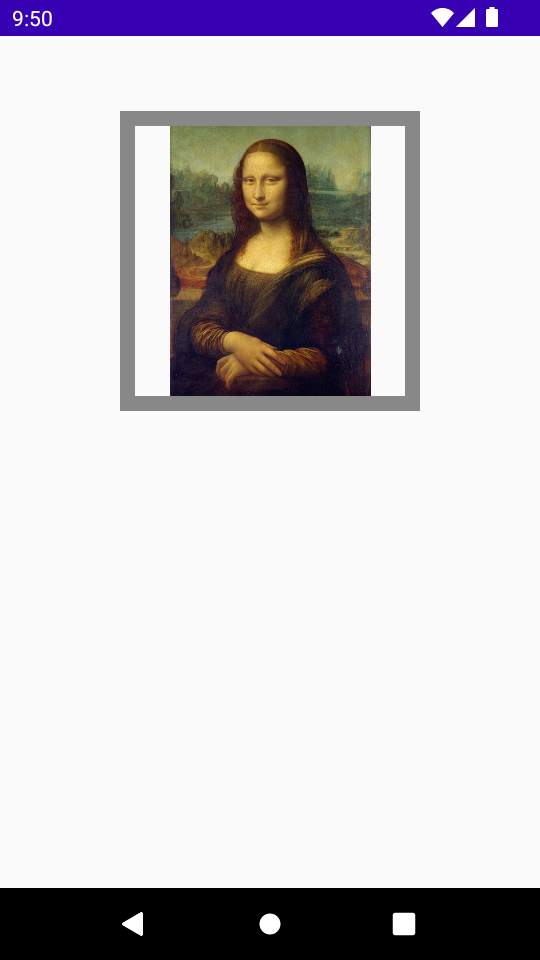
Scale Image – FillHeight
ContentScale.FillHeight
value scales the source image maintaining the aspect ratio so that the bounds match the available height for the Image composable.
Copy and replace the Image composable code in the MainActivity.kt with the following code.
Code for Image Composable
Image( painter = painterResource(id = R.drawable.monalisa), contentDescription = null, contentScale = ContentScale.FillHeight, modifier = Modifier .size(200.dp) .border( BorderStroke(10.dp, Color.Gray), RectangleShape ) )
Screenshot
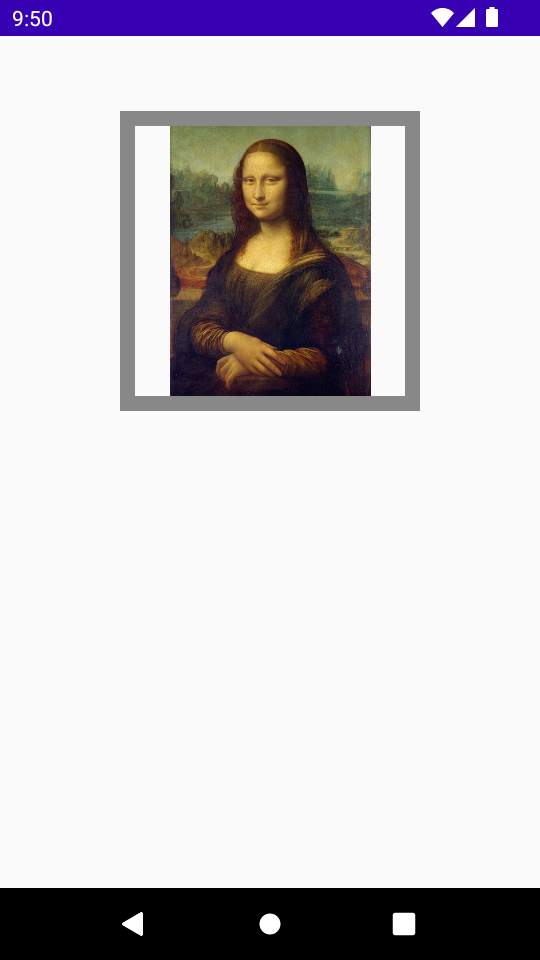
Scale Image – FillWidth
ContentScale.FillWidth
value scales the source image maintaining the aspect ratio so that the bounds match the available width for the Image composable.
Copy and replace the Image composable code in the MainActivity.kt with the following code.
Code for Image Composable
Image( painter = painterResource(id = R.drawable.monalisa), contentDescription = null, contentScale = ContentScale.FillWidth, modifier = Modifier .size(200.dp) .border( BorderStroke(10.dp, Color.Gray), RectangleShape ) )
Screenshot
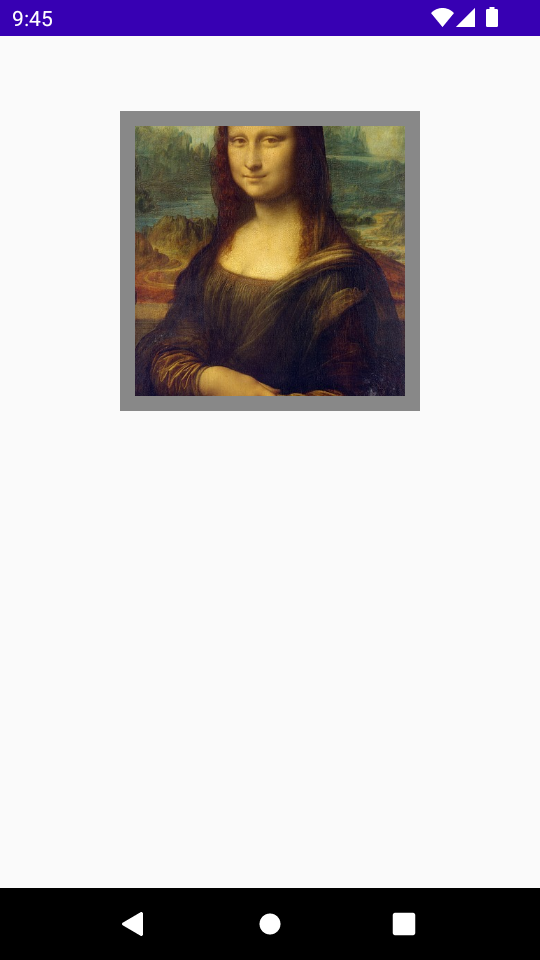
Scale Image – FillBounds
ContentScale.FillBounds
value scales the source image vertically and horizontally non-uniformly to fill the available space for the Image composable.
This type of scaling distorts the image if you place them in containers that do not match the exact ratio of the image.
Copy and replace the Image composable code in the MainActivity.kt with the following code.
Code for Image Composable
Image( painter = painterResource(id = R.drawable.monalisa), contentDescription = null, contentScale = ContentScale.FillBounds, modifier = Modifier .size(200.dp) .border( BorderStroke(10.dp, Color.Gray), RectangleShape ) )
Screenshot
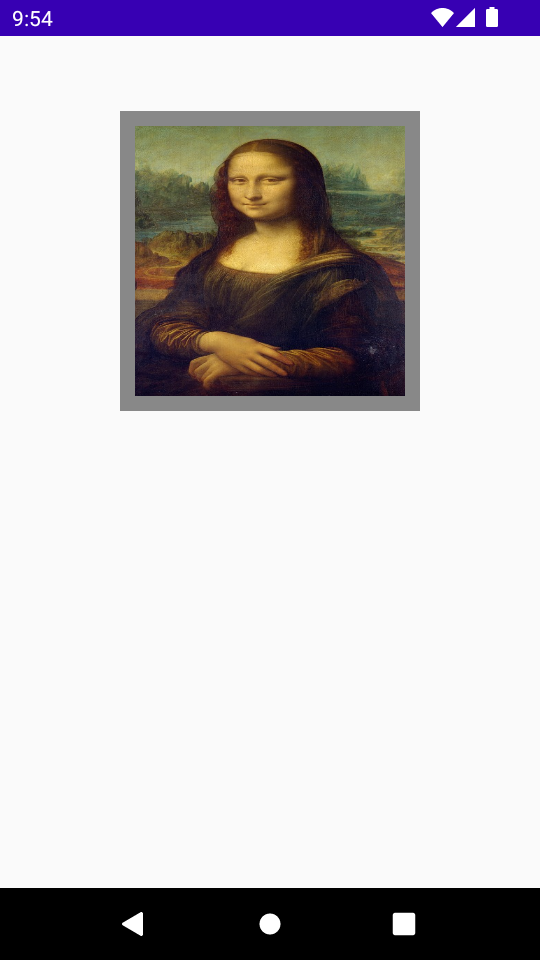
Scale Image – Inside
ContentScale.Inside
value scales the source image to maintain the aspect ratio inside the destination bounds.
If the source image is smaller than or equal to the destination in both dimensions, it behaves similarly to `None`.
Copy and replace the Image composable code in the MainActivity.kt with the following code.
Code for Image Composable
Image( painter = painterResource(id = R.drawable.monalisa), contentDescription = null, contentScale = ContentScale.Inside, modifier = Modifier .size(200.dp) .border( BorderStroke(10.dp, Color.Gray), RectangleShape ) )
Screenshot
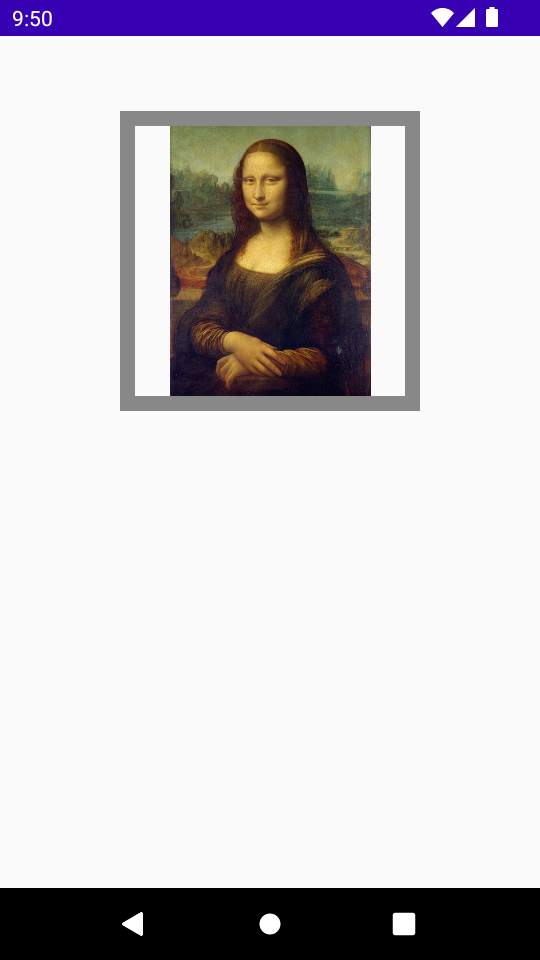
Scale Image – None
ContentScale.None
value does not apply any scaling to the source.
If the source image is smaller than available space for Image composable, the source image won’t be scaled up to fit the available area.
Copy and replace the Image composable code in the MainActivity.kt with the following code.
Code for Image Composable
Image( painter = painterResource(id = R.drawable.monalisa), contentDescription = null, contentScale = ContentScale.None, modifier = Modifier .size(200.dp) .border( BorderStroke(10.dp, Color.Gray), RectangleShape ) )
Screenshot
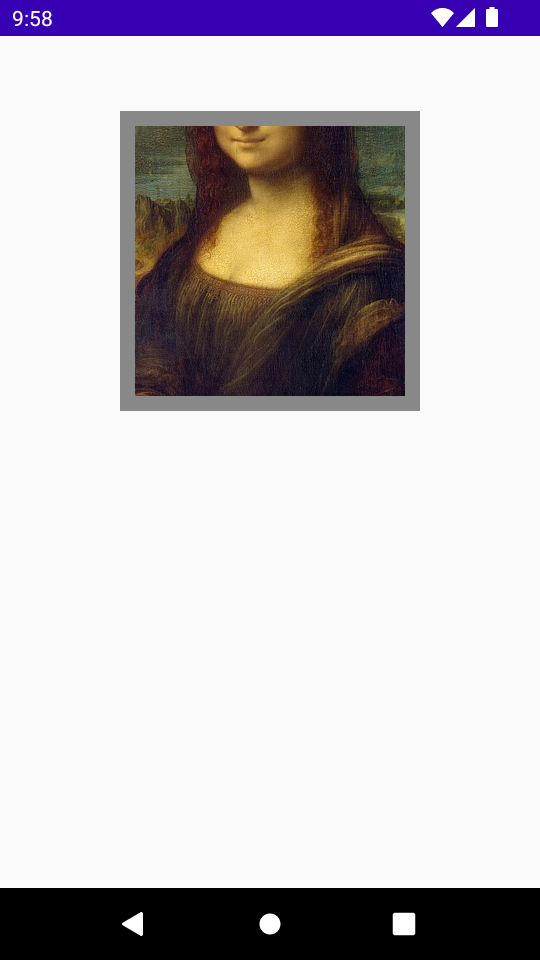
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to scale an image in Image composable.