Android Compose – Change Text Font Size
To change font size of Text composable in Android Jetpack Compose, pass a required font size value for the optional fontSize
parameter of Text composable.
import androidx.compose.ui.unit.sp Text( "Hello World", fontSize = 30.sp )
Make sure to import sp
, as shown in the above code snippet, when you are assign fontSize property with scale-independent pixels.
Example
Create an Android Application with Jetpack Compose as template, and modify MainActivity.kt as shown in the following.
The Text composable in the following activity displays Hello World
text and we shall set its font size to 30 scale-independent pixels is 30.sp
.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.compose.setContent import androidx.appcompat.app.AppCompatActivity import androidx.compose.foundation.layout.Column import androidx.compose.foundation.layout.fillMaxWidth import androidx.compose.material.Text import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.unit.sp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth() ) { Text( "Hello World", fontSize = 30.sp ) } } } } }
Screenshot in Emulator
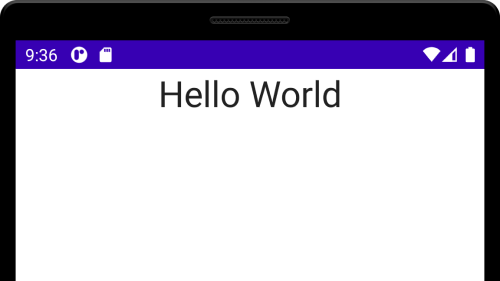
Let us define two Text composables, with font sizes 30sp and 50sp respectively, and observe the result.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.compose.setContent import androidx.appcompat.app.AppCompatActivity import androidx.compose.foundation.layout.Column import androidx.compose.foundation.layout.fillMaxWidth import androidx.compose.material.Text import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.unit.sp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth() ) { Text( "Hello World", fontSize = 30.sp ) Text( "Hello World", fontSize = 50.sp ) } } } } }
Screenshot in Emulator
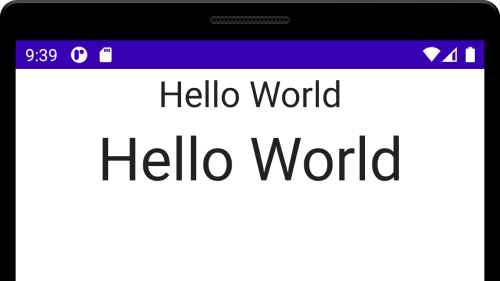
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to change the font size of Text composable in Android Project with Jetpack Compose.