Android Compose – Button Text Color
In this tutorial, we will learn how to set the color of text in a Button in Android Compose.
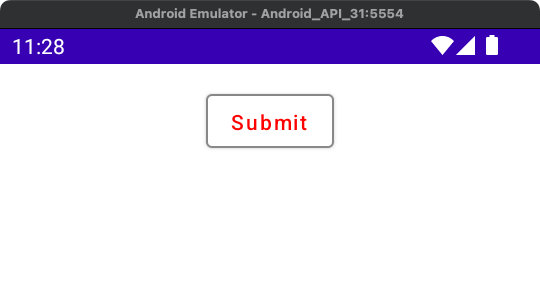
To set color of text in a Button in Android Jetpack Compose, we can directly set a color for the Text composable UI component that is used to display the content in the Button.
Button(
onClick = {},
) {
Text("Submit",
color = Color.Red,
)
}
Example
In this example, we have UI with a Button. The button has the text Submit
. And we set red color for the text in this Button.
Create a Project in Android Studio with empty compose activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
</>
Copy
package com.example.myapplication
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.BorderStroke
import androidx.compose.foundation.layout.*
import androidx.compose.material.Button
import androidx.compose.material.ButtonDefaults
import androidx.compose.material.Text
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.platform.LocalContext
import androidx.compose.ui.unit.dp
import com.example.myapplication.ui.theme.MyApplicationTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
Column(
horizontalAlignment = Alignment.CenterHorizontally,
modifier = Modifier
.padding(20.dp)
.fillMaxWidth()) {
Button(
border = BorderStroke(1.dp, Color.Gray),
colors = ButtonDefaults.outlinedButtonColors(backgroundColor = Color.White),
onClick = {},
) {
Text("Submit",
color = Color.Red,
)
}
}
}
}
}
}
Screenshot
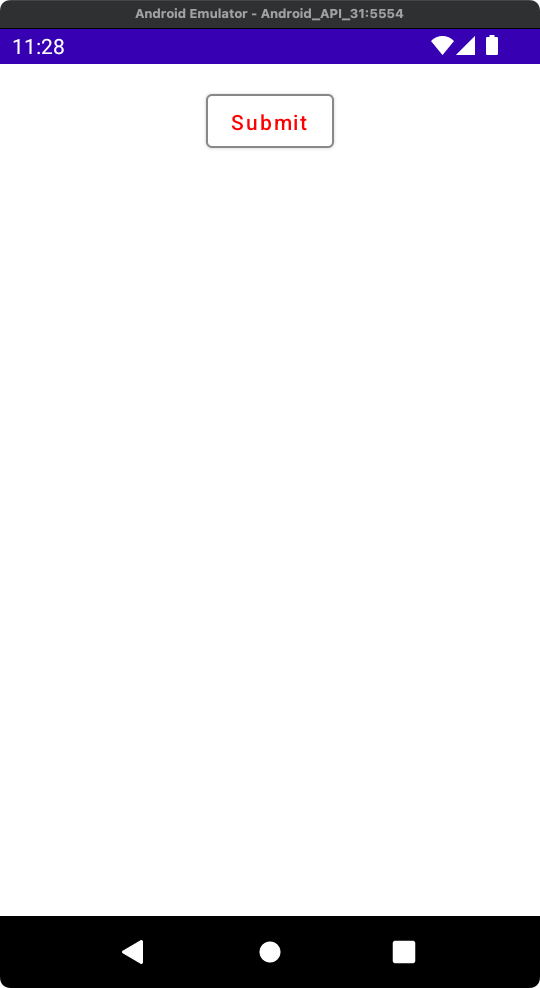
Black color text in button
Now, let us change the text color in the Button to black.
Code for Button
</>
Copy
Button(
border = BorderStroke(1.dp, Color.Gray),
colors = ButtonDefaults.outlinedButtonColors(backgroundColor = Color.White),
onClick = {},
) {
Text("Submit",
color = Color.Black
)
}
Screenshot
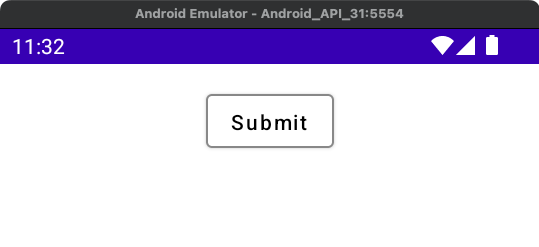
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set color for text in a Button.