Android Jetpack Compose – Set Background Color for Card
To set the background color for Card in Android Jetpack Compose, set backgroundColor
parameter with the required Color value.
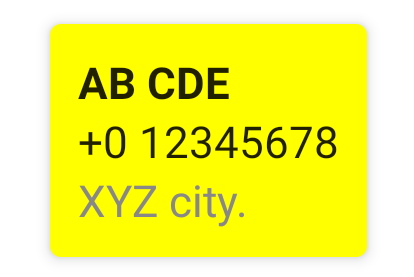
ADVERTISEMENT
Example
In this example, we shall display a Card composable and set its background color with Yellow.
Create a Project in Android Studio with Empty Compose Activity template, and modify MainActivity.kt file as shown in the following.
MainActivity.kt
package com.example.myapplication import android.os.Bundle import androidx.activity.compose.setContent import androidx.appcompat.app.AppCompatActivity import androidx.compose.foundation.layout.Column import androidx.compose.foundation.layout.fillMaxHeight import androidx.compose.foundation.layout.fillMaxWidth import androidx.compose.foundation.layout.padding import androidx.compose.material.Card import androidx.compose.material.Text import androidx.compose.ui.Alignment import androidx.compose.ui.Modifier import androidx.compose.ui.graphics.Color import androidx.compose.ui.text.font.FontWeight import androidx.compose.ui.unit.dp import com.example.myapplication.ui.theme.MyApplicationTheme class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContent { MyApplicationTheme { Column( horizontalAlignment = Alignment.CenterHorizontally, modifier = Modifier.fillMaxWidth().fillMaxHeight().padding(10.dp)) { Card( elevation = 4.dp, backgroundColor = Color.Yellow ) { Column(modifier = Modifier.padding(10.dp)) { Text("AB CDE", fontWeight = FontWeight.W700) Text("+0 12345678") Text("XYZ city.", color = Color.Gray) } } } } } } }
Screenshot
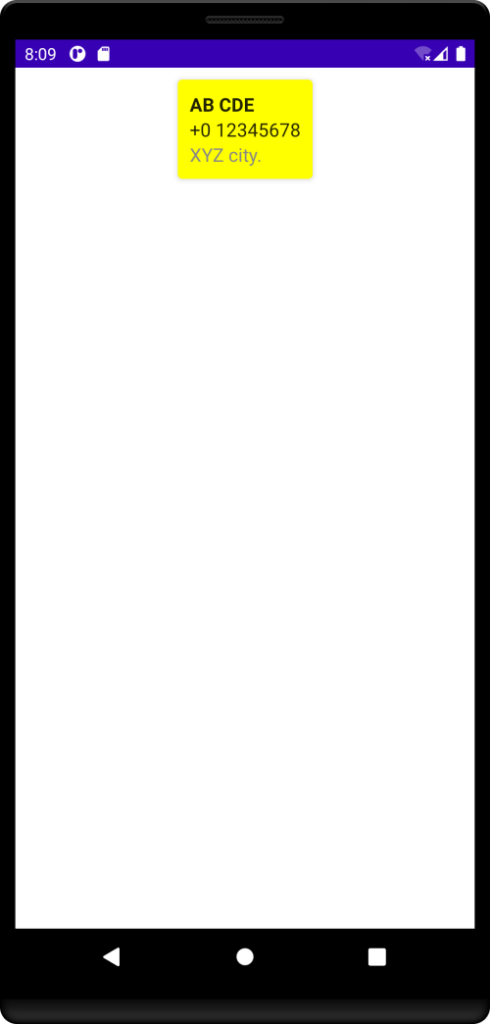
Conclusion
In this Android Jetpack Compose Tutorial, we learned how to set the background color for Card composable in Android Jetpack Compose.